在taro中开发小程序后端返回 900e8a75b40e62917e0125ba0dc255fc9972b0d63e53e42999ef0a6a92be4804前端如何渲染二维码并实现预览功能和上一张下一张功能
时间: 2023-12-10 16:38:14 浏览: 97
可以使用第三方库 `qrcode.react` 来生成二维码,然后利用 `Image` 组件来展示二维码图片。具体实现步骤如下:
1. 安装 `qrcode.react` 库:
```
npm install qrcode.react
```
2. 引入库文件:
```javascript
import QRCode from 'qrcode.react';
```
3. 在需要展示二维码的组件中,使用 `QRCode` 组件来生成二维码图片:
```javascript
<QRCode value="900e8a75b40e62917e0125ba0dc255fc9972b0d63e53e42999ef0a6a92be4804" />
```
`value` 属性指定了二维码的内容。
4. 添加上一张下一张功能:
可以使用 `state` 来存储当前二维码的索引,然后在上一张和下一张按钮的点击事件中更新索引并重新渲染二维码。
```javascript
class QRCodeGallery extends React.Component {
constructor(props) {
super(props);
this.state = {
index: 0, // 当前二维码的索引
codes: [
'900e8a75b40e62917e0125ba0dc255fc9972b0d63e53e42999ef0a6a92be4804',
'...',
'...'
]
};
}
handlePrevious = () => {
const index = Math.max(0, this.state.index - 1);
this.setState({ index });
};
handleNext = () => {
const index = Math.min(this.state.codes.length - 1, this.state.index + 1);
this.setState({ index });
};
render() {
const { index, codes } = this.state;
return (
<div>
<QRCode value={codes[index]} />
<button onClick={this.handlePrevious}>上一张</button>
<button onClick={this.handleNext}>下一张</button>
</div>
);
}
}
```
5. 添加预览功能:
可以使用 `react-modal` 库来实现弹窗预览。具体实现步骤如下:
- 安装 `react-modal` 库:
```
npm install react-modal
```
- 引入库文件:
```javascript
import Modal from 'react-modal';
```
- 在需要预览二维码的组件中,添加一个按钮,并在按钮的点击事件中设置弹窗的可见性和当前二维码的索引:
```javascript
class QRCodeGallery extends React.Component {
constructor(props) {
super(props);
this.state = {
index: 0, // 当前二维码的索引
codes: [
'900e8a75b40e62917e0125ba0dc255fc9972b0d63e53e42999ef0a6a92be4804',
'...',
'...'
],
isModalOpen: false // 弹窗的可见性
};
}
handlePreview = index => {
this.setState({ index, isModalOpen: true });
};
handleCloseModal = () => {
this.setState({ isModalOpen: false });
};
render() {
const { index, codes, isModalOpen } = this.state;
return (
<div>
<QRCode value={codes[index]} />
<button onClick={() => this.handlePreview(index)}>预览</button>
<Modal isOpen={isModalOpen} onRequestClose={this.handleCloseModal}>
<QRCode value={codes[index]} />
</Modal>
</div>
);
}
}
```
弹窗的可见性由 `isModalOpen` 控制,点击预览按钮时,调用 `handlePreview` 方法设置当前二维码的索引和弹窗的可见性为 `true`,点击弹窗的关闭按钮时,调用 `handleCloseModal` 方法设置弹窗的可见性为 `false`。在弹窗中展示当前二维码的内容即可。
阅读全文
相关推荐
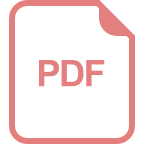
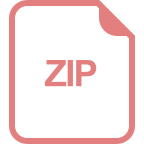
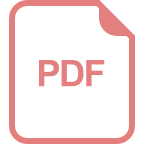
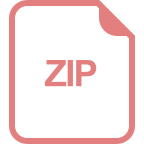
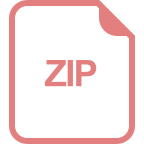
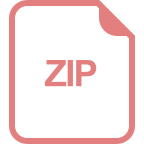






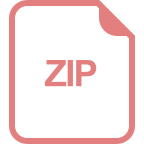
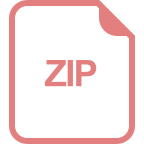
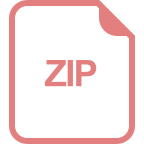
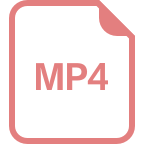
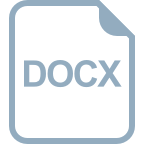
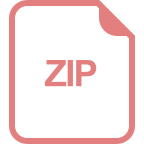