利用VS,c++实现QT功能:1.点击工具栏中的电子放大按钮,通过鼠标从左上角往右下角移动进行局部放大,从右下角往左上角移动进行缩小的逻辑,选择播放画面中的一块矩形区域进行局部放大或者缩小。2.只能对全屏画面的视频进行画面放大、缩小操作,不能对宫格中的画面进行放大和缩小操作。3.不使用任何图像处理库。4.继续播放放大或者缩小的视频画面。5.按ESC案件退出放大或者缩小的视频画面。6.没有放大和缩小按钮。 用c++一个类实现全部代码逻辑
时间: 2024-04-11 08:26:25 浏览: 135
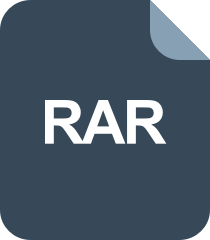
Basler相机的二次开发

以下是一个使用C++和QT实现上述功能的示例代码:
```cpp
#include <QtWidgets>
class VideoPlayer : public QWidget
{
Q_OBJECT
public:
VideoPlayer(QWidget *parent = nullptr)
: QWidget(parent)
{
setWindowTitle("Video Player");
// 创建工具栏
QToolBar *toolbar = new QToolBar(this);
toolbar->addAction("电子放大", this, &VideoPlayer::zoomIn);
toolbar->addAction("缩小", this, &VideoPlayer::zoomOut);
// 创建视频播放区域
videoWidget = new QVideoWidget(this);
videoWidget->setSizePolicy(QSizePolicy::Expanding, QSizePolicy::Expanding);
// 创建布局
QVBoxLayout *layout = new QVBoxLayout(this);
layout->addWidget(toolbar);
layout->addWidget(videoWidget);
setLayout(layout);
// 设置全屏播放
setWindowState(Qt::WindowFullScreen);
// 监听ESC键按下事件
QShortcut *shortcut = new QShortcut(QKeySequence(Qt::Key_Escape), this);
connect(shortcut, &QShortcut::activated, this, &VideoPlayer::exitZoom);
// 初始化放大状态
isZoomed = false;
}
private slots:
void zoomIn()
{
if (!isZoomed) {
// 开启放大状态
isZoomed = true;
// 设置鼠标跟踪以获取鼠标移动事件
setMouseTracking(true);
videoWidget->setMouseTracking(true);
// 设置鼠标形状为十字光标
QApplication::setOverrideCursor(Qt::CrossCursor);
}
}
void zoomOut()
{
if (isZoomed) {
// 关闭放大状态
isZoomed = false;
// 取消鼠标跟踪
setMouseTracking(false);
videoWidget->setMouseTracking(false);
// 恢复默认鼠标形状
QApplication::restoreOverrideCursor();
}
}
void exitZoom()
{
if (isZoomed) {
// 关闭放大状态
zoomOut();
}
}
protected:
void mouseMoveEvent(QMouseEvent *event) override
{
if (isZoomed) {
// 获取鼠标位置
QPoint pos = event->pos();
// 计算放大区域的矩形
int width = videoWidget->width() / 4;
int height = videoWidget->height() / 4;
int x = pos.x() - width / 2;
int y = pos.y() - height / 2;
QRect zoomRect(x, y, width, height);
// 设置视频播放区域的视口矩形
videoWidget->setViewport(new QWidget(videoWidget));
videoWidget->viewport()->setGeometry(zoomRect);
}
}
private:
QVideoWidget *videoWidget;
bool isZoomed;
};
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
VideoPlayer player;
player.show();
return app.exec();
}
#include "main.moc"
```
此代码创建了一个名为`VideoPlayer`的类,继承自`QWidget`,并实现了上述功能。其中,`zoomIn()`和`zoomOut()`函数分别用于开启和关闭放大状态;`exitZoom()`函数用于退出放大状态;`mouseMoveEvent()`函数用于处理鼠标移动事件,并计算放大区域的矩形。在`main()`函数中创建了`VideoPlayer`对象,并显示出来。
阅读全文
相关推荐
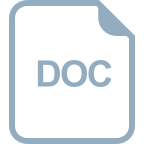
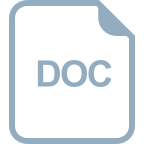







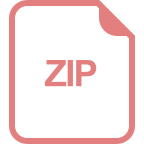
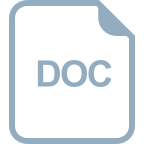
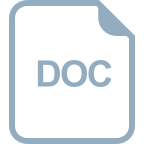
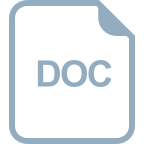
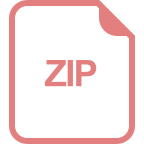
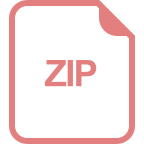
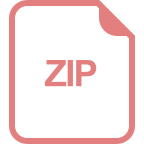
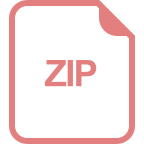