// 发件人电子邮箱 String from = "from@example.com"; // 收件人电子邮箱 String to = "to@example.com"; // 指定发送邮件的主机为 smtp.qq.com String host = "smtp.qq.com"; //QQ 邮件服务器 // 获取系统属性 Properties properties = System.getProperties(); // 设置邮件服务器 properties.setProperty("mail.smtp.host", host); properties.put("mail.smtp.auth", "true"); // 获取默认session对象 Session session = Session.getDefaultInstance(properties, new Authenticator(){ public java.net.PasswordAuthentication getPasswordAuthentication() { return new PasswordAuthentication("你的QQ账号", from); //发件人邮件用户名、密码 } }); 这部分内容进行修改优化 以springboot框架
时间: 2024-02-15 14:12:22 浏览: 23
好的,以下是使用 Spring Boot 框架发送邮件的示例代码:
首先,需要在 pom.xml 文件中添加依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-mail</artifactId>
</dependency>
```
然后,在 application.properties 文件中添加邮件相关的配置:
```properties
# 邮件服务器主机名
spring.mail.host=smtp.qq.com
# 邮件服务器端口号
spring.mail.port=587
# 邮件服务器用户名
spring.mail.username=your_qq_email_account
# 邮件服务器密码或授权码
spring.mail.password=your_qq_email_password_or_auth_code
# 开启 TLS 加密传输
spring.mail.properties.mail.smtp.starttls.enable=true
# 邮件编码格式
spring.mail.default-encoding=UTF-8
```
然后,可以定义一个 MailService 类来发送邮件:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.mail.SimpleMailMessage;
import org.springframework.mail.javamail.JavaMailSender;
import org.springframework.stereotype.Service;
@Service
public class MailService {
@Autowired
private JavaMailSender mailSender;
public void sendSimpleMail(String to, String subject, String content) {
SimpleMailMessage message = new SimpleMailMessage();
message.setTo(to);
message.setSubject(subject);
message.setText(content);
mailSender.send(message);
}
}
```
在这个示例中,我们使用 Spring Boot 自带的 JavaMailSender 来发送邮件。可以看到,发送邮件只需要调用 sendSimpleMail 方法,并指定收件人、主题和内容即可。
希望这可以帮助您在 Spring Boot 中发送邮件。
相关推荐
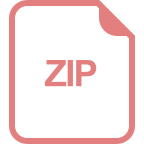














