java web实现商品添加代码
时间: 2023-11-26 12:06:28 浏览: 80
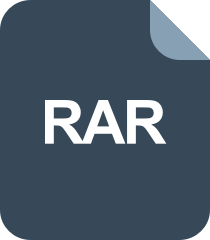
商品添加代码
以下是Java Web实现商品添加的代码示例:
1. 创建商品表的SQL语句
```
CREATE TABLE `product` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`name` varchar(255) NOT NULL,
`description` varchar(255) DEFAULT NULL,
`price` decimal(10,2) NOT NULL,
`stock` int(11) NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=1 DEFAULT CHARSET=utf8;
```
2. 创建商品添加页面
在HTML中创建一个表单,包含商品名称、商品描述、价格、库存等表单项,表单的action属性指向商品添加处理程序的URL。
```
<form action="/product/add" method="post">
<label for="name">商品名称:</label>
<input type="text" id="name" name="name"><br>
<label for="description">商品描述:</label>
<textarea id="description" name="description"></textarea><br>
<label for="price">商品价格:</label>
<input type="text" id="price" name="price"><br>
<label for="stock">商品库存:</label>
<input type="text" id="stock" name="stock"><br>
<input type="submit" value="添加">
</form>
```
3. 创建商品添加处理程序
在Java Web框架中创建一个Controller,接收来自前端页面的POST请求,将表单数据解析并保存到数据库中。
```
@Controller
@RequestMapping("/product")
public class ProductController {
@Autowired
private ProductService productService;
@PostMapping("/add")
public String addProduct(@RequestParam("name") String name,
@RequestParam("description") String description,
@RequestParam("price") BigDecimal price,
@RequestParam("stock") Integer stock) {
Product product = new Product();
product.setName(name);
product.setDescription(description);
product.setPrice(price);
product.setStock(stock);
productService.addProduct(product);
return "redirect:/product/list";
}
}
```
4. 实现商品列表页面
在HTML中创建一个表格,展示所有已添加的商品信息,其中每一行都包含商品ID、商品名称、商品描述、价格、库存等信息。
```
<table>
<thead>
<tr>
<th>ID</th>
<th>名称</th>
<th>描述</th>
<th>价格</th>
<th>库存</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<tr th:each="product : ${products}">
<td th:text="${product.id}"></td>
<td th:text="${product.name}"></td>
<td th:text="${product.description}"></td>
<td th:text="${product.price}"></td>
<td th:text="${product.stock}"></td>
<td>
<a th:href="@{/product/edit/{id}(id=${product.id})}">编辑</a>
<a th:href="@{/product/delete/{id}(id=${product.id})}">删除</a>
</td>
</tr>
</tbody>
</table>
```
5. 实现商品编辑和删除功能
在Java Web框架中创建商品编辑处理程序和商品删除处理程序,分别用于处理编辑和删除操作。
```
@Controller
@RequestMapping("/product")
public class ProductController {
@Autowired
private ProductService productService;
@GetMapping("/edit/{id}")
public String editProduct(@PathVariable("id") Long id, Model model) {
Product product = productService.getProductById(id);
model.addAttribute("product", product);
return "edit";
}
@PostMapping("/update")
public String updateProduct(@ModelAttribute Product product) {
productService.updateProduct(product);
return "redirect:/product/list";
}
@GetMapping("/delete/{id}")
public String deleteProduct(@PathVariable("id") Long id) {
productService.deleteProduct(id);
return "redirect:/product/list";
}
}
```
以上就是Java Web实现商品添加的代码示例,其中ProductService和ProductDao的实现需要根据具体框架和数据库进行相应的调整。
阅读全文
相关推荐
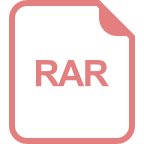
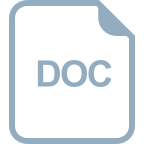
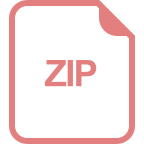
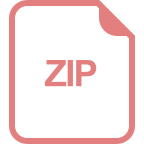
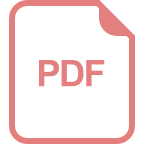
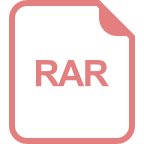
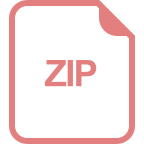
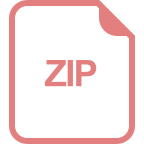
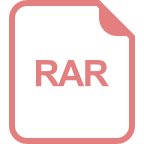
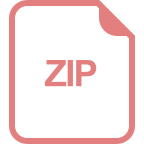
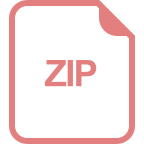
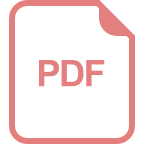
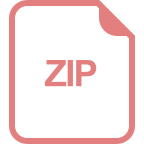
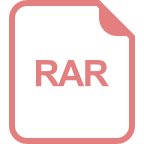
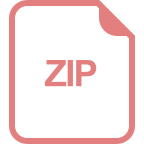