请示用aop的方式,并且将日志保存到D:/code/logs/coursemanagement.log文件中
时间: 2024-01-05 20:02:55 浏览: 87
好的,下面是使用AOP和Java Spring Boot将日志保存到文件的代码示例:
1. 首先,我们需要添加依赖,如下所示:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-aop</artifactId>
</dependency>
<dependency>
<groupId>org.apache.logging.log4j</groupId>
<artifactId>log4j-slf4j-impl</artifactId>
<version>2.14.0</version>
</dependency>
```
其中,`spring-boot-starter-aop`是Spring Boot的AOP依赖,`log4j-slf4j-impl`是Log4j的SLF4J适配器,这里我们使用Log4j来实现日志的文件输出。
2. 然后,我们需要在`application.properties`中添加Log4j的配置,如下所示:
```properties
logging.level.root=info
logging.file.path=D:/code/logs
logging.file.name=coursemanagement.log
logging.pattern.console=%d{yyyy-MM-dd HH:mm:ss.SSS} [%thread] %-5level %logger{36} - %msg%n
logging.pattern.file=%d{yyyy-MM-dd HH:mm:ss.SSS} [%thread] %-5level %logger{36} - %msg%n
```
这里我们将日志级别设为`info`,并指定日志文件的路径和文件名,日志输出的格式也可以自定义,这里我们将日志输出到控制台和文件中。
3. 接下来,我们需要定义一个切面类,如下所示:
```java
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.annotation.AfterThrowing;
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Before;
import org.springframework.stereotype.Component;
@Aspect
@Component
public class LoggingAspect {
private static final Logger logger = LogManager.getLogger(LoggingAspect.class);
@Before("execution(* com.example.coursemanagement.controller.*.*(..))")
public void logBefore(JoinPoint joinPoint) {
logger.info("Entering {}.{}() with arguments {}",
joinPoint.getSignature().getDeclaringTypeName(),
joinPoint.getSignature().getName(),
joinPoint.getArgs());
}
@AfterThrowing(pointcut = "execution(* com.example.coursemanagement.controller.*.*(..))", throwing = "ex")
public void logAfterThrowing(JoinPoint joinPoint, Throwable ex) {
logger.error("Exception in {}.{}() with cause = {}",
joinPoint.getSignature().getDeclaringTypeName(),
joinPoint.getSignature().getName(),
ex.getCause() != null ? ex.getCause() : "NULL");
}
}
```
在上面的代码中,我们定义了一个名为`LoggingAspect`的切面类,在类上使用了`@Aspect`和`@Component`注解。
在类中,我们定义了两个切面方法,分别是`logBefore`和`logAfterThrowing`,分别用于记录方法进入和方法异常信息。
其中,我们使用了Log4j的`LogManager`和`Logger`类来定义日志记录器,使用了`@Before`和`@AfterThrowing`注解来定义切面方法的类型和切入点,使用`joinPoint`参数来获取方法签名和参数信息,最后使用日志记录器来输出日志信息。
4. 最后,我们需要在`application.properties`中指定日志输出的类型为`file`,如下所示:
```properties
logging.type=file
```
这样,日志信息就可以输出到指定路径下的文件中了。
希望这段代码可以帮助你更好地理解使用AOP和Java Spring Boot将日志保存到文件中的方法。
阅读全文
相关推荐
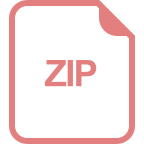
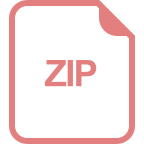
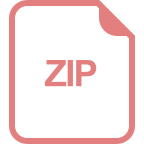
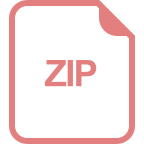
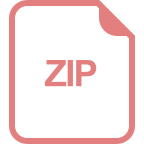
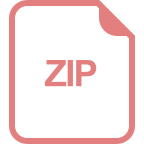
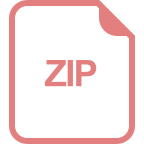
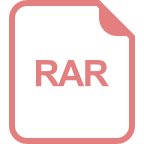
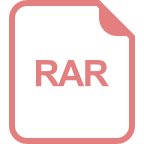
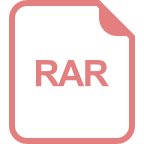
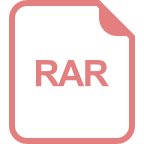
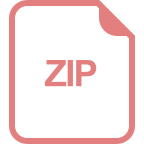
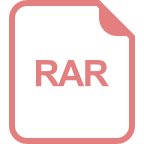
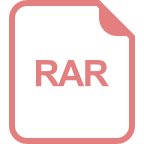
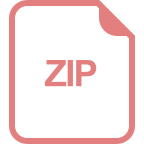
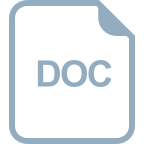