对一个m行n列的列表沿着行进行RTS平滑处理
时间: 2024-04-11 10:28:11 浏览: 24
要对一个m行n列的列表沿着行进行RTS平滑处理,可以使用循环或者`np.apply_along_axis`函数来逐行处理。下面是使用循环的示例代码:
```python
import numpy as np
def rts_smooth(data, window_size, smooth_factor):
assert window_size % 2 == 1, "Window size must be odd"
assert 0 <= smooth_factor <= 1, "Smooth factor must be between 0 and 1"
m, n = data.shape
half_window = (window_size - 1) // 2
smoothed_data = np.zeros_like(data)
for i in range(m):
row = data[i]
for j in range(half_window, n - half_window):
window = row[j - half_window:j + half_window + 1]
median = np.median(window)
deviation = np.abs(window - median)
threshold = smooth_factor * np.median(deviation)
if np.abs(row[j] - median) > threshold:
smoothed_data[i, j] = median
else:
smoothed_data[i, j] = row[j]
smoothed_data[i, :half_window] = row[:half_window]
smoothed_data[i, n - half_window:] = row[n - half_window:]
return smoothed_data
# 使用示例
data = np.array([[10, 15, 20, 12, 18, 22, 25, 16, 14, 23],
[5, 8, 10, 12, 9, 7, 15, 20, 18, 17],
[2, 4, 6, 8, 10, 12, 14, 16, 18, 20]])
window_size = 3
smooth_factor = 0.6
smoothed_data = rts_smooth(data, window_size, smooth_factor)
print(smoothed_data)
```
这样,`rts_smooth`函数将会对输入数据的每一行进行平滑处理,输出结果将保持与输入数据相同的形状。每行的数据都会被独立处理。
相关推荐
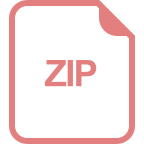
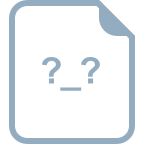
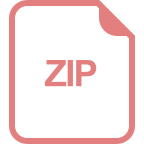








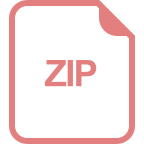
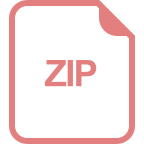
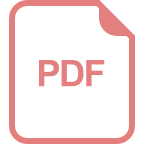
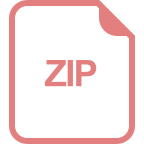
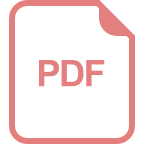