Reason: groovy.lang.MissingMethodException: No signature of method: static com.github.difflib.DiffUtils.htmlDiff() is applicable for argument types: (com.github.difflib.patch.Patch) values: [Patch{deltas=[[ChangeDelta, position: 3, lines: ["013","Smith","25","Smith@email.com", "013","Son","25","Son@email.com"] to ["015","Smith","25","Smith@email.com", "020","Son","25","Son@email.com", "021","Amy","018","Amy@email.com"]]]}]
时间: 2023-07-03 15:25:49 浏览: 41
这个错误提示是因为在java-diff-utils 4.7版本中,htmlDiff()方法已经被移除了,需要使用DiffRowGenerator类来自定义差异结果的输出格式。
下面是一个修改后的脚本,使用DiffRowGenerator类来生成差异结果的HTML格式。其中,自定义了添加和删除行的样式,并使用了Java 8的Stream API来进行处理。
```groovy
@Grab(group='com.github.java-diff-utils', module='diffutils', version='4.7')
import difflib.*
import java.nio.file.*
import java.util.concurrent.*
// 文件夹1和文件夹2的路径
def folder1Path = Paths.get("folder1")
def folder2Path = Paths.get("folder2")
// 并行流线程池
def pool = Executors.newFixedThreadPool(Runtime.getRuntime().availableProcessors())
// 定义差异结果的HTML样式
def addedStyle = "background-color: #cfc;"
def deletedStyle = "background-color: #fcc;"
// 自定义DiffRowGenerator类,生成差异结果的HTML格式
class MyDiffRowGenerator extends DiffRowGenerator.Builder {
MyDiffRowGenerator() {
showInlineDiffs(false)
ignoreWhiteSpaces(true)
oldTag((line) -> {
if (line == null) {
return null
} else {
return "<span class='deleted'>" + line + "</span>"
}
})
newTag((line) -> {
if (line == null) {
return null
} else {
return "<span class='added'>" + line + "</span>"
}
})
}
}.build()
// 比较文件夹1和文件夹2中的所有文件
def results = Files.walk(folder1Path)
.parallel()
.filter { Files.isRegularFile(it) }
.map { file1 ->
def file2 = folder2Path.resolve(folder1Path.relativize(file1))
if (Files.exists(file2)) {
// 文件在两个文件夹中都存在
def content1 = Files.readAllLines(file1)
def content2 = Files.readAllLines(file2)
if (content1 == content2) {
[file: file1, result: "文件内容相同"]
} else {
def patch = DiffUtils.diff(content1, content2)
def rows = MyDiffRowGenerator().generateDiffRows(content1, content2, patch)
def diffHtml = rows.stream().map { row ->
if (row.tag == Tag.INSERT) {
"<span class='added'>" + row.text + "</span>"
} else if (row.tag == Tag.DELETE) {
"<span class='deleted'>" + row.text + "</span>"
} else {
row.text
}
}.collect(Collectors.joining("<br>"))
[file: file1, result: diffHtml]
}
} else {
// 文件在一个文件夹中存在但在另一个文件夹中不存在
[file: file1, result: "文件不存在"]
}
}
.collect { it }
// 将比较结果以HTML格式呈现
def html = new StringBuilder()
html.append("<html><head><style>")
html.append(".added { $addedStyle }")
html.append(".deleted { $deletedStyle }")
html.append("</style></head><body>")
results.each { result ->
html.append("<h2>").append(result.file).append("</h2>")
if (result.result instanceof String) {
html.append("<p>").append(result.result).append("</p>")
} else {
html.append(result.result)
}
}
html.append("</body></html>")
println(html)
```
这个脚本会遍历文件夹1中的所有文件,与文件夹2中的对应文件进行比较,并将比较结果以HTML格式输出。如果文件在两个文件夹中都存在但内容不同,则将差异以HTML格式呈现,并将差异字段高亮显示。如果文件在一个文件夹中存在但在另一个文件夹中不存在,则将其标记为“文件不存在”。并行流可以提高性能,使得比较更快完成。
相关推荐
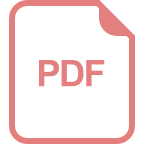
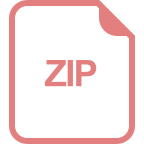















