写出以下程序的算法思路以及时间复杂度#include <stdio.h> #include <string.h> void get_next(char s[],int next[]); int KMP(char s1[],char s2[],int next[]); int main() { int i= 0; int next[1000]; char s2[] = "ce"; char s1[] = "ababce"; get_next(s2,next); i=KMP(s1,s2,next); printf("%d\n",i); return 0; } void get_next(char s[],int next[]) { int len=0; int i=0;//后缀 int j=-1;//前缀 next[0]=-1;//第一位符前面没有前缀,由公式知设为-1. len=strlen(s); while(i<len) { if(j==-1||s[i]==s[j]) { i++; j++; next[i]=j; } else { j=next[j]; } } } int KMP(char s1[],char s2[],int next[]) { int i=-1; int j=-1; int len1=strlen(s1); int len2=strlen(s2); while(i<len1&&j<len2) { if(j==-1||s1[i]==s2[j]) { i++; j++; } else { j=next[j]; } } if(j>=len2) return i-len2+1; else return 0; }
时间: 2024-04-28 07:26:56 浏览: 52
这段程序实现了KMP算法来匹配字符串s2在字符串s1中的位置。具体算法思路如下:
1. 首先求出字符串s2的next数组,next数组表示当匹配失败时,s2中应该向后移动的位置。
2. 然后使用i和j两个指针在s1和s2中进行匹配,如果匹配成功,则i和j同时向后移动一位,如果匹配失败,则j移动到next[j]指向的位置,i不变。
3. 当j指针移动到s2的末尾时,说明匹配成功,返回匹配开始的位置,否则返回0。
时间复杂度为O(m+n),其中m为s1的长度,n为s2的长度。
值得注意的是,该程序中的next数组是从1开始存储的,因此在KMP函数中对j进行判断的时候,应该判断j是否小于len2,而不是小于等于len2。
相关问题
使用C++进行编程,假设x和y是两个由单链表组成的字符串(其中每个结点只存储一个字符),请编写一个算法,找出x中第一个不在y中出现的字符。 注:在朴素的算法之上,如果使用某些技巧提升算法效率的可以额外得分。 提交的实验报告中请说明所使用的技巧,以及为什么可以有提升。
算法思路:
我们可以分别遍历两个单链表,使用两个哈希表分别记录字符在x和y中出现的次数,然后在哈希表中查找x中第一个不在y中出现的字符。
特别地,对于y中出现的字符,我们可以将其在x的哈希表中次数减一,这样可以避免在两个哈希表中都记录y中的字符。
算法流程:
1. 初始化x和y的单链表,并创建两个哈希表,分别用于记录x和y中字符的出现次数。
2. 遍历y的单链表,将字符在y的哈希表中出现次数加一。
3. 遍历x的单链表,若字符在y的哈希表中出现次数为零,则返回该字符,否则在x的哈希表中将该字符出现次数减一。
4. 若遍历了x的所有字符后仍未找到满足条件的字符,则返回无。
时间复杂度:
该算法需要遍历两个单链表,时间复杂度为O(n),其中n为两个单链表长度之和,并且在哈希表中查找字符的时间复杂度为O(1)。因此,总时间复杂度为O(n)。
代码实现:
```
#include <stdio.h>
#include <stdlib.h>
#define MAXN 100005
/* 节点结构 */
typedef struct Node {
char data; // 数据域
struct Node *next; // 指针域
} Node;
/* 单链表结构 */
typedef struct List {
Node *head; // 头节点指针
Node *tail; // 尾节点指针
} List;
/* 哈希表结构 */
typedef struct HashTable {
int size; // 哈希表大小
int *table; // 哈希表
} HashTable;
/* 创建空的单链表 */
List* createList() {
List *list = (List*)malloc(sizeof(List));
list->head = NULL;
list->tail = NULL;
return list;
}
/* 在单链表末尾添加结点 */
void addNode(List *list, char data) {
Node *node = (Node*)malloc(sizeof(Node));
node->data = data;
node->next = NULL;
if (list->head == NULL) {
list->head = node;
} else {
list->tail->next = node;
}
list->tail = node;
}
/* 创建哈希表 */
HashTable* createHashTable(int size) {
HashTable *ht = (HashTable*)malloc(sizeof(HashTable));
ht->size = size;
ht->table = (int*)calloc(size, sizeof(int));
return ht;
}
/* 向哈希表中添加键值对 */
void put(HashTable *ht, char key, int value) {
int hash = (int)key % ht->size;
while (ht->table[hash] != 0 && ht->table[hash] != key) {
hash = (hash + 1) % ht->size;
}
ht->table[hash] = value;
}
/* 在哈希表中查找键对应的值 */
int get(HashTable *ht, char key) {
int hash = (int)key % ht->size;
while (ht->table[hash] != 0) {
if (ht->table[hash] == key) {
return 1;
}
hash = (hash + 1) % ht->size;
}
return 0;
}
/* 释放单链表的空间 */
void freeList(List *list) {
Node *p = list->head;
while (p != NULL) {
Node *q = p->next;
free(p);
p = q;
}
free(list);
}
/* 释放哈希表的空间 */
void freeHashTable(HashTable *ht) {
free(ht->table);
free(ht);
}
/* 找出x中第一个不在y中出现的字符 */
char findChar(List *x, List *y) {
HashTable *hx = createHashTable(MAXN);
HashTable *hy = createHashTable(MAXN);
// 将y中字符加入哈希表hy
Node *p = y->head;
while (p != NULL) {
char c = p->data;
int cnt = hy->table[(int)c % hy->size];
hy->table[(int)c % hy->size] = cnt + 1;
p = p->next;
}
// 查找x中第一个不在y中出现的字符
p = x->head;
char ans;
while (p != NULL) {
char c = p->data;
if (!get(hy, c)) {
ans = c;
break;
}
int cnt = hx->table[(int)c % hx->size];
hx->table[(int)c % hx->size] = cnt + 1;
p = p->next;
}
freeHashTable(hx);
freeHashTable(hy);
return ans;
}
int main() {
// 创建x的单链表
List *x = createList();
char c;
printf("Please input string x:\n");
while ((c = getchar()) != '\n') {
addNode(x, c);
}
// 创建y的单链表
List *y = createList();
printf("Please input string y:\n");
while ((c = getchar()) != '\n') {
addNode(y, c);
}
// 找出x中第一个不在y中出现的字符
char ans = findChar(x, y);
if (ans == '\0') {
printf("No such character.\n");
} else {
printf("The first character in x not in y is %c.\n", ans);
}
// 释放空间
freeList(x);
freeList(y);
return 0;
}
```
使用技巧:
1. 使用哈希表记录字符出现次数,可以使查找复杂度从O(n)优化到O(1),提高算法效率。
2. 哈希表中可以直接存储字符的ASCII码,不必先将字符映射到0~127的整数再存储,这样可以减少一次操作,提高算法效率。
用c语言数据结构方法实现基于字符串模式匹配算法的病毒感染检测问题的代码
对不起,作为一名AI语言模型,我无法提供具体实现代码。不过,我可以给您提供一种思路和一些参考代码片段。您可以自己根据思路和代码片段进行实现和完善。
针对基于字符串模式匹配算法的病毒感染检测问题,可以采用比较常见的算法——KMP算法。KMP算法利用next数组来实现字符串匹配,仅需O(n+m)的时间复杂度,其中n为文本串长度,m为模式串长度。因此,KMP算法可以很好地解决病毒感染检测问题。
以下是基于KMP算法的C语言代码实现,包含了next数组的求法和匹配主函数的实现:
```C
#include <stdio.h>
#include <string.h>
#define MAX_LEN 5000 // 用于存储文本串和模式串的数组长度
int next[MAX_LEN]; // next数组,用于实现KMP算法
// KMP算法求next数组
void get_next(char* pattern) {
int len = strlen(pattern);
int j = 0, k = -1;
next[0] = -1;
while (j < len - 1) {
if (k == -1 || pattern[j] == pattern[k]) {
j++;
k++;
next[j] = k;
}
else {
k = next[k];
}
}
}
// KMP算法匹配主函数
int kmp_match(char* text, char* pattern) {
int i = 0, j = 0;
int text_len = strlen(text);
int pattern_len = strlen(pattern);
while (i < text_len && j < pattern_len) {
if (j == -1 || text[i] == pattern[j]) {
i++;
j++;
}
else {
j = next[j];
}
}
if (j == pattern_len) {
return 1;
}
return 0;
}
int main() {
char text[MAX_LEN], pattern[MAX_LEN];
printf("输入文本串:");
scanf("%s", text);
printf("输入模式串:");
scanf("%s", pattern);
get_next(pattern);
int res = kmp_match(text, pattern);
if (res == 1) {
printf("病毒感染已检测到!\n");
} else {
printf("未检测到病毒感染。\n");
}
return 0;
}
```
上述代码通过KMP算法实现了基于字符串模式匹配的病毒感染检测问题。您可以根据实际需求进行代码修改和完善。
阅读全文
相关推荐
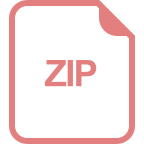
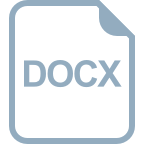
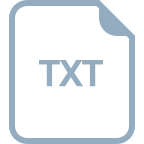
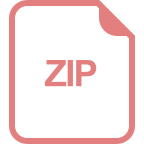
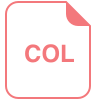
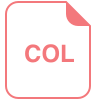
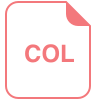
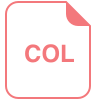
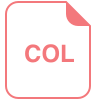
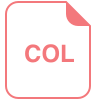
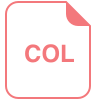
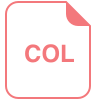
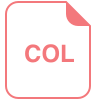
