接口怎么使用netty框架
时间: 2023-06-09 14:05:31 浏览: 96
ChitGPT并不具备技术实现的能力,它只是一个模型,可以通过为其提供输入来调用它并生成相应的输出。因此,ChitGPT并不会提供任何接口来使用netty框架。在您的情况下,您可以通过使用netty框架来开发自己的应用程序,并与ChitGPT进行集成。具体实现详情您可以参考相关的技术文档,或者在CSDN社区中寻找相关的技术资源。
相关问题
jar rpc netty框架
jar rpc netty框架是一种基于Java语言开发的轻量级RPC(远程过程调用)框架,它使用Netty作为底层通信框架,提供了高性能、高可靠性、可扩展性、易于使用的RPC服务。
使用jar rpc netty框架,开发人员可以非常简单地实现分布式系统中的服务调用。在使用过程中,开发人员只需要定义接口、实现服务,并使用框架提供的注解和配置即可完成服务注册和发现等工作。框架还提供了负载均衡、超时控制、自动重试等功能,可以满足大部分分布式系统的需求。
总的来说,jar rpc netty框架是一个非常优秀的RPC框架,可以帮助开发人员快速、简单地构建高性能、高可靠性的分布式系统。
java rpc netty框架代码
以下是一个基于Netty框架的简单Java RPC实现,包含服务端和客户端的代码:
服务端代码:
```java
public class RpcServer {
private final EventLoopGroup bossGroup;
private final EventLoopGroup workerGroup;
public RpcServer() {
bossGroup = new NioEventLoopGroup();
workerGroup = new NioEventLoopGroup();
}
public void start(int port) throws InterruptedException {
try {
ServerBootstrap bootstrap = new ServerBootstrap();
bootstrap.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
protected void initChannel(SocketChannel ch) throws Exception {
ChannelPipeline pipeline = ch.pipeline();
pipeline.addLast(new ObjectDecoder(ClassResolvers.cacheDisabled(null)));
pipeline.addLast(new ObjectEncoder());
pipeline.addLast(new RpcServerHandler());
}
})
.option(ChannelOption.SO_BACKLOG, 128)
.childOption(ChannelOption.SO_KEEPALIVE, true);
ChannelFuture future = bootstrap.bind(port).sync();
System.out.println("Server started on port " + port);
future.channel().closeFuture().sync();
} finally {
workerGroup.shutdownGracefully();
bossGroup.shutdownGracefully();
}
}
}
```
客户端代码:
```java
public class RpcClient {
private final EventLoopGroup group;
private final Bootstrap bootstrap;
public RpcClient() {
group = new NioEventLoopGroup();
bootstrap = new Bootstrap();
bootstrap.group(group)
.channel(NioSocketChannel.class)
.handler(new ChannelInitializer<SocketChannel>() {
@Override
protected void initChannel(SocketChannel ch) throws Exception {
ChannelPipeline pipeline = ch.pipeline();
pipeline.addLast(new ObjectDecoder(ClassResolvers.cacheDisabled(null)));
pipeline.addLast(new ObjectEncoder());
pipeline.addLast(new RpcClientHandler());
}
});
}
public Object send(String host, int port, Object request) throws InterruptedException, ExecutionException {
try {
ChannelFuture future = bootstrap.connect(host, port).sync();
RpcClientHandler handler = future.channel().pipeline().get(RpcClientHandler.class);
handler.setRequest(request);
return handler.getResponse();
} finally {
group.shutdownGracefully();
}
}
}
```
服务端处理请求的代码:
```java
public class RpcServerHandler extends SimpleChannelInboundHandler<RpcRequest> {
@Override
protected void channelRead0(ChannelHandlerContext ctx, RpcRequest request) throws Exception {
String className = request.getClassName();
Class<?> clazz = Class.forName(className);
Object service = BeanUtils.getBean(clazz);
Method method = service.getClass().getMethod(request.getMethodName(), request.getParameterTypes());
Object result = method.invoke(service, request.getParameters());
ctx.writeAndFlush(result);
}
}
```
客户端发送请求的代码:
```java
public class RpcClientHandler extends SimpleChannelInboundHandler<Object> {
private Object request;
private Object response;
public void setRequest(Object request) {
this.request = request;
}
public Object getResponse() {
return response;
}
@Override
public void channelActive(ChannelHandlerContext ctx) throws Exception {
ctx.writeAndFlush(request);
}
@Override
protected void channelRead0(ChannelHandlerContext ctx, Object msg) throws Exception {
response = msg;
ctx.close();
}
}
```
以上代码实现了一个简单的RPC框架,可以在服务端注册服务并提供接口,客户端可以通过接口调用远程服务并获取结果。需要注意的是,这个实现并没有考虑很多实际应用中需要考虑的问题,如服务的负载均衡、故障转移、超时处理等等,仅作为一个初步的参考。
阅读全文
相关推荐
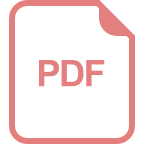
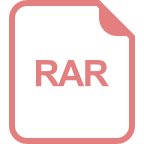
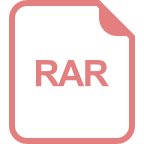
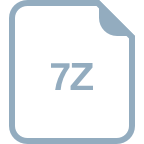
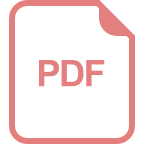
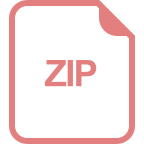
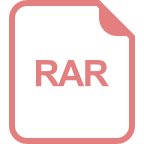
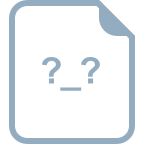
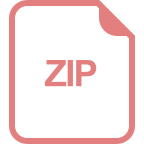
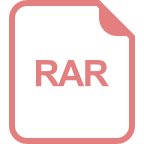
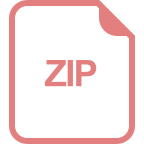
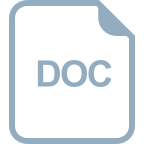
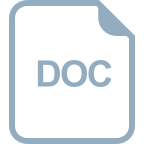
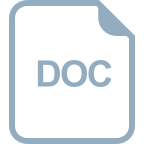
