使用Csharp写一个多个双线程的代码
时间: 2023-01-15 20:08:23 浏览: 87
下面是一个用 C# 实现多个双线程的示例代码:
```
using System;
using System.Threading;
namespace MultiThreadingExample
{
class Program
{
static void Main(string[] args)
{
// 创建 5 个线程
for (int i = 0; i < 5; i++)
{
Thread thread = new Thread(ThreadMethod);
thread.Start();
}
Console.ReadLine();
}
static void ThreadMethod()
{
// 打印当前线程的线程号
Console.WriteLine($"当前线程的线程号为: {Thread.CurrentThread.ManagedThreadId}");
}
}
}
```
上述代码会创建 5 个线程,并打印每个线程的线程号。
注意:如果需要使用双线程(即每个核心有两个线程),你需要使用 `System.Threading.Tasks.Parallel` 命名空间下的 `Parallel.For` 或 `Parallel.ForEach` 方法。这些方法可以并行地执行循环。
下面是使用 `Parallel.For` 实现的双线程版本:
```
using System;
using System.Threading.Tasks;
namespace MultiThreadingExample
{
class Program
{
static void Main(string[] args)
{
// 创建 5 个双线程(即 10 个线程)
Parallel.For(0, 5, i =>
{
ThreadMethod();
ThreadMethod();
});
Console.ReadLine();
}
static void ThreadMethod()
{
// 打印当前线程的线程号
Console.WriteLine($"当前线程的线程号为: {Thread.CurrentThread.ManagedThreadId}");
}
}
}
```
相关推荐
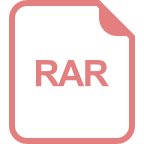














