如何用VS的C++语言写一个学生实验课选课系统
时间: 2023-12-10 18:03:27 浏览: 74
首先,你需要设计一个学生实验课选课系统的数据模型,例如:学生信息、实验课程信息、选课信息等。然后,你可以使用C++语言中的面向对象编程思想来实现这个系统。
以下是一个简单的实现步骤:
1. 定义学生类和实验课程类,包括属性和方法。
2. 定义选课类,包括学生和实验课程的信息,以及选课操作等方法。
3. 实现主函数,创建学生和实验课程对象,并进行选课操作。
4. 将数据存储到文件中,以便下次启动程序时可以读取上一次的选课信息。
以下是一个简单的代码示例:
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
using namespace std;
class Student {
public:
Student(string name, string major, int id) {
this->name = name;
this->major = major;
this->id = id;
}
string name;
string major;
int id;
};
class Course {
public:
Course(string name, int id, int capacity) {
this->name = name;
this->id = id;
this->capacity = capacity;
}
string name;
int id;
int capacity;
};
class Enrollment {
public:
Enrollment(Student student, Course course) {
this->student = student;
this->course = course;
}
Student student;
Course course;
};
void save(vector<Enrollment>& enrollments) {
ofstream file("enrollments.txt");
for (auto enrollment : enrollments) {
file << enrollment.student.name << ",";
file << enrollment.student.major << ",";
file << enrollment.student.id << ",";
file << enrollment.course.name << ",";
file << enrollment.course.id << ",";
file << enrollment.course.capacity << "\n";
}
file.close();
}
vector<Enrollment> load() {
vector<Enrollment> enrollments;
ifstream file("enrollments.txt");
if (file.is_open()) {
string line;
while (getline(file, line)) {
string name, major, courseName;
int id, courseId, capacity;
int index = 0;
for (int i = 0; i < line.size(); i++) {
if (line[i] == ',') {
string field = line.substr(index, i - index);
index = i + 1;
if (name.empty()) {
name = field;
} else if (major.empty()) {
major = field;
} else if (id == 0) {
id = stoi(field);
} else if (courseName.empty()) {
courseName = field;
} else if (courseId == 0) {
courseId = stoi(field);
} else if (capacity == 0) {
capacity = stoi(field);
}
}
}
enrollments.push_back(Enrollment(Student(name, major, id), Course(courseName, courseId, capacity)));
}
file.close();
}
return enrollments;
}
int main() {
vector<Enrollment> enrollments = load();
Student alice("Alice", "Computer Science", 1001);
Student bob("Bob", "Mathematics", 1002);
Course calculus("Calculus", 2001, 30);
Course programming("Programming", 2002, 20);
enrollments.push_back(Enrollment(alice, calculus));
enrollments.push_back(Enrollment(bob, programming));
save(enrollments);
return 0;
}
```
在上面的示例中,我们定义了学生、实验课程和选课类,并实现了数据存储和加载。在主函数中,我们创建了两个学生和两门实验课程,并进行选课操作。最后,我们将选课信息存储到文件中,并在下次启动程序时加载上一次的选课信息。
阅读全文
相关推荐






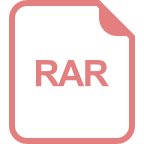








