splitContainer1.Panel2 自动计算所选遥感影像边界,并且绘制
时间: 2024-01-20 10:02:57 浏览: 32
要自动计算所选遥感影像的边界,并在 `splitContainer1.Panel2` 控件中绘制边界框,您需要使用 GDAL 库来读取遥感影像的元数据,获取图像的范围,然后将其转换为控件上的坐标系。在 `splitContainer1_Panel2_Paint` 函数中,我们可以绘制边界框。
以下是示例代码:
```csharp
private string imagePath; // 遥感影像路径
private RectangleF imageBounds; // 遥感影像边界框
private void openButton_Click(object sender, EventArgs e)
{
// 打开遥感影像文件并计算边界框
OpenFileDialog openFileDialog = new OpenFileDialog();
if (openFileDialog.ShowDialog() == DialogResult.OK)
{
// 保存遥感影像路径
imagePath = openFileDialog.FileName;
// 使用 GDAL 库读取遥感影像元数据
Gdal.AllRegister();
Dataset dataset = Gdal.Open(imagePath, Access.GA_ReadOnly);
double[] geoTransform = new double[6];
dataset.GetGeoTransform(geoTransform);
// 计算遥感影像边界框
int imageWidth = dataset.RasterXSize;
int imageHeight = dataset.RasterYSize;
double minX = geoTransform[0];
double minY = geoTransform[3] + geoTransform[5] * imageHeight;
double maxX = geoTransform[0] + geoTransform[1] * imageWidth;
double maxY = geoTransform[3];
imageBounds = new RectangleF((float)minX, (float)minY, (float)(maxX - minX), (float)(maxY - maxY));
// 刷新控件
splitContainer1.Panel2.Invalidate();
}
}
private void splitContainer1_Panel2_Paint(object sender, PaintEventArgs e)
{
// 绘制遥感影像边界框
if (!string.IsNullOrEmpty(imagePath))
{
// 计算遥感影像边界框在控件中的位置和大小
int imageWidth = splitContainer1.Panel2.Width;
int imageHeight = splitContainer1.Panel2.Height;
float scaleX = imageWidth / imageBounds.Width;
float scaleY = imageHeight / imageBounds.Height;
float scale = Math.Min(scaleX, scaleY);
float imageX = -imageBounds.X * scale;
float imageY = -imageBounds.Y * scale;
float imageWidthScaled = imageBounds.Width * scale;
float imageHeightScaled = imageBounds.Height * scale;
// 绘制遥感影像边界框
using (Pen pen = new Pen(Color.Red, 2))
{
e.Graphics.DrawRectangle(pen, imageX, imageY, imageWidthScaled, imageHeightScaled);
}
}
}
```
在上面的代码中,我们首先定义了一个 `imagePath` 变量和一个 `imageBounds` 变量,用于保存遥感影像的路径和边界框。在 `openButton_Click` 函数中,我们使用 `OpenFileDialog` 对象打开一个遥感影像文件,并使用 GDAL 库读取其元数据。然后,我们计算遥感影像的边界框,并将其保存到 `imageBounds` 变量中。最后,我们调用 `splitContainer1.Panel2.Invalidate` 方法刷新控件,从而触发 `splitContainer1_Panel2_Paint` 函数重新绘制控件。
在 `splitContainer1_Panel2_Paint` 函数中,我们首先检查 `imagePath` 变量是否为空,如果为空,则不绘制边界框。否则,我们计算遥感影像边界框在控件中的位置和大小,并使用 `Graphics` 对象的 `DrawRectangle` 方法绘制边界框。
请注意,使用 GDAL 库读取遥感影像的元数据可能需要一定的时间,具体取决于图像的大小和计算机的性能。如果您需要处理大型遥感影像,请考虑使用后台线程来读取元数据。另外,请确保在程序结束时释放 GDAL 库和遥感影像资源。
相关推荐
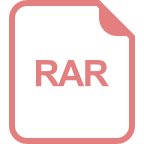














