python RGB图像旋转任何角度且没有黑边的代码
时间: 2023-08-31 14:30:45 浏览: 98
### 回答1:
下面是一个 Python 实现的 RGB 图像旋转函数,可以旋转任意角度且不会出现黑边:
```python
import numpy as np
from scipy import ndimage
def rotate_image(image, angle):
# 将角度转换为弧度
angle = np.deg2rad(angle)
# 计算旋转后的图像大小
width, height = image.shape[:2]
new_width = int(np.round(abs(width * np.cos(angle)) + abs(height * np.sin(angle))))
new_height = int(np.round(abs(height * np.cos(angle)) + abs(width * np.sin(angle))))
# 计算旋转后的中心点
cx, cy = width / 2, height / 2
new_cx, new_cy = new_width / 2, new_height / 2
# 构建旋转矩阵
rotation_matrix = np.array([
[np.cos(angle), -np.sin(angle), (1 - np.cos(angle)) * cx + np.sin(angle) * cy],
[np.sin(angle), np.cos(angle), (1 - np.cos(angle)) * cy - np.sin(angle) * cx],
[0, 0, 1]
])
# 应用旋转变换
rotated_image = ndimage.interpolation.affine_transform(
image,
rotation_matrix[:2, :2],
offset=rotation_matrix[:2, 2],
output_shape=(new_height, new_width),
order=3,
mode='constant',
cval=0
)
return rotated_image.astype(image.dtype)
```
这个函数使用了 `numpy` 和 `scipy` 库中的函数。它将输入图像旋转给定的角度,而且不会出现黑边。
### 回答2:
在Python中,可以使用PIL库(Python Imaging Library)来实现旋转RGB图像且没有黑边的效果。以下是一个示例代码:
```python
from PIL import Image
def rotate_image(image_path, angle):
# 打开图像
image = Image.open(image_path)
# 获得旋转后的图像
rotated_image = image.rotate(angle, expand=True)
# 创建一个新图像,大小与旋转后图像相同,背景为白色
new_image = Image.new("RGB", rotated_image.size, "white")
# 计算新图像的位置,使得旋转后图像居中显示
x = int((rotated_image.size[0] - image.size[0]) / 2)
y = int((rotated_image.size[1] - image.size[1]) / 2)
# 将旋转后的图片粘贴到新图像中
new_image.paste(rotated_image, (x, y))
# 显示或保存旋转后的图像
new_image.show()
# new_image.save("rotated_image.jpg")
# 调用函数进行旋转
rotate_image('image.jpg', 30)
```
这段代码首先使用`Image.open()`打开输入图像,并使用`rotate()`函数对图像进行旋转,`angle`是旋转角度。设置`expand=True`参数可以确保旋转后的图像不会被裁剪。
接下来,根据旋转后图像的尺寸,创建一个新的图像对象(背景为白色),并计算旋转后图像与新图像的位置偏移量,确保旋转后的图像居中显示。
最后,使用`paste()`函数将旋转后的图像粘贴到新的图像对象中,然后可以选择显示或保存旋转后的图像。在上述代码中,使用`show()`函数来显示旋转后的图像,如果需要保存旋转后的图像,可以使用`save()`函数。
以上代码可以实现对RGB图像进行任意角度的旋转,旋转后的图像会居中显示,不会出现黑边。
### 回答3:
可以使用OpenCV库来实现Python RGB图像旋转任何角度且没有黑边的代码。下面是一个简单的示例代码:
```
import cv2
import numpy as np
def rotate_image(image, angle):
# 获取图像的宽度和高度
height, width = image.shape[:2]
# 构造旋转矩阵
rotation_matrix = cv2.getRotationMatrix2D((width/2, height/2), angle, 1)
# 计算新图像的宽度和高度
new_width = int(np.ceil(width * abs(np.cos(np.radians(angle)))) + np.ceil(height * abs(np.sin(np.radians(angle))))))
new_height = int(np.ceil(height * abs(np.cos(np.radians(angle)))) + np.ceil(width * abs(np.sin(np.radians(angle))))))
# 调整旋转矩阵的平移分量,以确保图像在旋转后仍然完全可见
rotation_matrix[0, 2] += (new_width - width) / 2
rotation_matrix[1, 2] += (new_height - height) / 2
# 执行旋转操作
rotated_image = cv2.warpAffine(image, rotation_matrix, (new_width, new_height))
return rotated_image
# 读取RGB图像
image = cv2.imread('input_image.jpg', cv2.IMREAD_COLOR)
# 设置旋转角度为45度
angle = 45
# 旋转图像
rotated_image = rotate_image(image, angle)
# 显示旋转后的图像
cv2.imshow('Rotated Image', rotated_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这个代码中,首先读取了一个RGB图像,然后定义了一个`rotate_image`函数用来旋转图像。这个函数使用`getRotationMatrix2D`来获取旋转矩阵,并且计算出新图像的宽度和高度。为了确保旋转后的图像没有黑边,还需要调整旋转矩阵的平移分量。最后,使用`warpAffine`函数执行实际的图像旋转操作。在示例中,旋转角度设置为45度,可以根据需求修改。最后,使用`imshow`函数显示旋转后的图像。
相关推荐
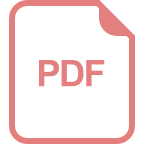
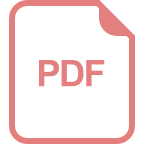
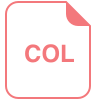













