请实现MyPreDate类,并设计测试用例对之进行测试。
时间: 2024-12-19 22:17:34 浏览: 10
为了实现MyPreDate类,我们可以首先定义类结构,包括上面提到的四个方法:
```java
import java.text.SimpleDateFormat;
import java.util.Date;
public class MyPreDate {
private SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
// 验证日期方法
public boolean validateDate(String inputDate) throws IllegalArgumentException {
try {
Date date = sdf.parse(inputDate);
return sdf.format(date).equals(inputDate); // 如果解析后再格式化结果不变,则日期有效
} catch (Exception e) {
return false;
}
}
// 判断是否为大月
public boolean isBigMonth(int month) {
switch (month) {
case 1:
case 3:
case 5:
case 7:
case 8:
case 10:
case 12:
return true;
default:
return false;
}
}
// 判断是否是闰年
public boolean isLeapYear(int year) {
return (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0);
}
// 输出前一天日期
public String preDate(String inputDate) throws IllegalArgumentException {
if (!validateDate(inputDate)) throw new IllegalArgumentException("Invalid date");
int year = Integer.parseInt(sdf.parse(inputDate).toString().substring(0, 4));
int month = Integer.parseInt(sdf.parse(inputDate).toString().substring(5, 7));
int day = Integer.parseInt(sdf.parse(inputDate).toString().substring(8));
if (isLeapYear(year) && month == 2) { // 跳过闰年的2月29日
day--;
} else if (isBigMonth(month)) {
day--;
} else {
throw new IllegalArgumentException("Cannot subtract a day from a non-existent date in a small month");
}
return sdf.format(new Date(year, month, day));
}
}
```
接下来是针对这个类的一些测试用例:
```java
import org.junit.Test;
import static org.junit.Assert.*;
public class MyPreDateTest {
@Test
public void testValidDate() {
MyPreDate myDate = new MyPreDate();
assertTrue(myDate.validateDate("2019-05-05"));
assertFalse(myDate.validateDate("2019-02-30")); // 这个日期不存在
}
@Test
public void testIsBigMonth() {
MyPreDate myDate = new MyPreDate();
assertEquals(true, myDate.isBigMonth(1)); // 一月
assertEquals(false, myDate.isBigMonth(2)); // 二月
}
@Test
public void testIsLeapYear() {
MyPreDate myDate = new MyPreDate();
assertEquals(true, myDate.isLeapYear(2020));
assertEquals(false, myDate.isLeapYear(2019));
}
@Test
public void testPreDate() {
MyPreDate myDate = new MyPreDate();
try {
assertEquals("2019-05-04", myDate.preDate("2019-05-05"));
} catch (IllegalArgumentException e) {
fail("An exception should not be thrown");
}
try {
assertEquals("2019-02-28", myDate.preDate("2019-03-01")); // 跳过闰年的2月29日
} catch (IllegalArgumentException e) {
fail("An exception should not be thrown");
}
try {
assertEquals("2019-04-30", myDate.preDate("2019-05-01")); // 平月减一天
} catch (IllegalArgumentException e) {
fail("An exception should not be thrown");
}
}
}
```
这些测试用例涵盖了类的不同方面,确保了各个方法的正常工作。
阅读全文
相关推荐



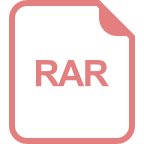
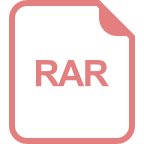

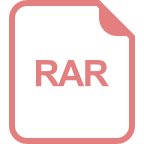

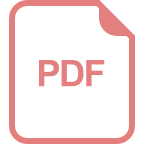
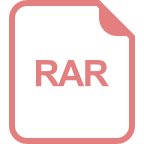




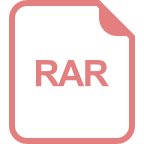


