用c++编写程序,创建包含10个数的数组,使用循环语句为数组赋值,输出其中最大值及对应的元素下标。考虑整型、小数、字符等不同类型数组的情况。 方法 函数模板原型: template<typename T> int getMaxIndex(T a[], int size=10);
时间: 2024-03-23 16:39:10 浏览: 96
好的,我可以回答这个问题。以下是对应的C++代码:
```cpp
#include <iostream>
using namespace std;
template<typename T>
int getMaxIndex(T a[], int size = 10) {
int maxIndex = 0;
for (int i = 1; i < size; i++) {
if (a[i] > a[maxIndex]) {
maxIndex = i;
}
}
return maxIndex;
}
int main() {
// 整型数组
int intArray[] = {5, 3, 2, 7, 8, 1, 6, 4, 9, 0};
int intMaxIndex = getMaxIndex(intArray);
cout << "整型数组最大值为:" << intArray[intMaxIndex] << ",下标为:" << intMaxIndex << endl;
// 小数数组
double doubleArray[] = {5.3, 3.2, 2.7, 7.8, 1.6, 4.9, 0.1, 6.4, 8.5, 9.7};
int doubleMaxIndex = getMaxIndex(doubleArray);
cout << "小数数组最大值为:" << doubleArray[doubleMaxIndex] << ",下标为:" << doubleMaxIndex << endl;
// 字符数组
char charArray[] = {'a', 'c', 'f', 'b', 'd', 'z', 'y', 'x', 'g', 'h'};
int charMaxIndex = getMaxIndex(charArray);
cout << "字符数组最大值为:" << charArray[charMaxIndex] << ",下标为:" << charMaxIndex << endl;
return 0;
}
```
该程序中使用了函数模板,可以处理不同类型的数组。在 `getMaxIndex` 函数中,遍历数组并记录最大值的下标,最后返回该下标即可。在 `main` 函数中,分别定义了整型、小数和字符数组,并分别调用 `getMaxIndex` 函数输出最大值和对应的下标。
阅读全文
相关推荐
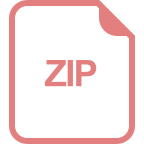
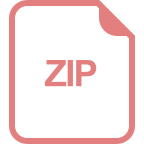















