winform电子相册源码
时间: 2023-08-30 20:11:45 浏览: 121
以下是一个简单的Winform电子相册源码示例,它可以浏览本地图片,并具有基本的图片操作功能(如旋转、放大/缩小等):
```csharp
using System;
using System.Drawing;
using System.IO;
using System.Windows.Forms;
namespace ElectronicPhotoAlbum
{
public partial class MainForm : Form
{
private int currentImageIndex = 0;
private string[] imagePaths;
public MainForm()
{
InitializeComponent();
}
private void MainForm_Load(object sender, EventArgs e)
{
// 获取本地图片路径
imagePaths = Directory.GetFiles(@"C:\MyImages", "*.jpg");
if (imagePaths.Length > 0)
{
// 显示第一张图片
ShowImage(currentImageIndex);
}
else
{
MessageBox.Show("No images found in the specified folder.");
Close();
}
}
private void ShowImage(int index)
{
if (index < 0 || index >= imagePaths.Length) return;
// 加载图片
Image image = Image.FromFile(imagePaths[index]);
// 显示图片
pictureBox.Image = image;
// 更新状态栏
toolStripStatusLabel.Text = $"Image {index + 1} of {imagePaths.Length}";
// 释放资源
image.Dispose();
}
private void btnPrevious_Click(object sender, EventArgs e)
{
// 显示上一张图片
currentImageIndex--;
if (currentImageIndex < 0) currentImageIndex = imagePaths.Length - 1;
ShowImage(currentImageIndex);
}
private void btnNext_Click(object sender, EventArgs e)
{
// 显示下一张图片
currentImageIndex++;
if (currentImageIndex >= imagePaths.Length) currentImageIndex = 0;
ShowImage(currentImageIndex);
}
private void btnRotateLeft_Click(object sender, EventArgs e)
{
// 左旋转图片
pictureBox.Image.RotateFlip(RotateFlipType.Rotate270FlipNone);
pictureBox.Invalidate();
}
private void btnRotateRight_Click(object sender, EventArgs e)
{
// 右旋转图片
pictureBox.Image.RotateFlip(RotateFlipType.Rotate90FlipNone);
pictureBox.Invalidate();
}
private void btnZoomIn_Click(object sender, EventArgs e)
{
// 放大图片
pictureBox.Width = (int)(pictureBox.Width * 1.1);
pictureBox.Height = (int)(pictureBox.Height * 1.1);
}
private void btnZoomOut_Click(object sender, EventArgs e)
{
// 缩小图片
pictureBox.Width = (int)(pictureBox.Width * 0.9);
pictureBox.Height = (int)(pictureBox.Height * 0.9);
}
}
}
```
使用方法:
1. 在Visual Studio中创建一个新的Winform应用程序项目。
2. 在窗体设计器中添加一个PictureBox控件和一些Button控件,用于浏览图片和执行操作。
3. 将上面的代码复制到Form1.cs文件中。
4. 根据需要修改代码,例如更改本地图片路径、更改按钮文本等。
5. 编译并运行程序,浏览本地图片并进行基本的图片操作。
阅读全文
相关推荐
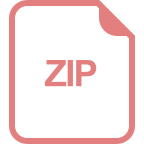
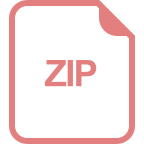
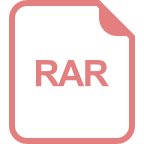
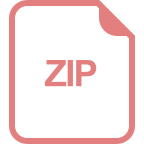
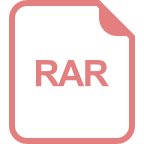
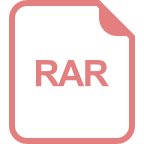
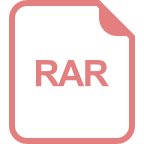
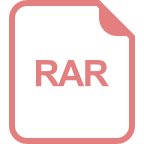
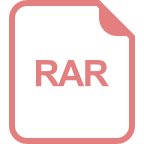
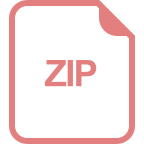
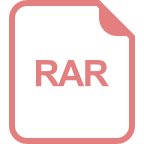
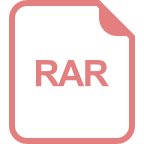
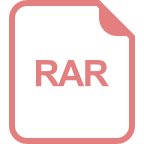
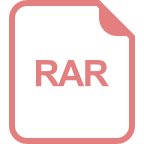
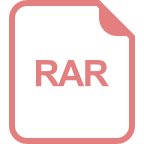