UE5.0.1 蓝图如何转出c++
时间: 2024-05-28 09:14:25 浏览: 197
在UE5中,可以使用以下步骤将蓝图转换为C++代码:
1. 打开需要转换的蓝图
2. 点击“菜单栏-蓝图编辑器-转换为C++类”
3. 在弹出的对话框中选择要保存的目录和文件名
4. 点击“保存”按钮
转换后的C++代码将自动生成并保存到指定的目录中。你可以在Visual Studio或其他C++编辑器中打开该文件,进行编辑和编译。请注意,转换后的C++代码可能需要手动添加一些逻辑以实现与蓝图相同的功能。
相关问题
ffmpeg 5.0.1版本用C++写一个在vs2019编译通过的rtsp播放器
好的,我可以为您提供一个简单的基于FFmpeg的RTSP播放器示例。
首先,您需要下载FFmpeg的最新版本,并将其添加到您的VS2019项目中。在项目属性中添加以下包含目录和库目录:
包含目录:`<FFmpeg目录>/include`
库目录:`<FFmpeg目录>/lib`
然后,您需要在项目中添加以下库文件:
```
avcodec.lib
avformat.lib
avutil.lib
swscale.lib
```
接下来,您可以使用以下代码创建一个简单的RTSP播放器:
```c++
#include <iostream>
#include <string>
#include <opencv2/opencv.hpp>
extern "C" {
#include <libavformat/avformat.h>
#include <libavcodec/avcodec.h>
#include <libavutil/imgutils.h>
#include <libswscale/swscale.h>
}
using namespace std;
using namespace cv;
int main(int argc, char* argv[])
{
av_register_all();
avformat_network_init();
AVFormatContext* pFormatCtx = nullptr;
AVCodecContext* pCodecCtx = nullptr;
AVCodec* pCodec = nullptr;
AVPacket* packet = nullptr;
int videoStream = -1;
int frameFinished = 0;
string url = "rtsp://<your_rtsp_url>";
if (avformat_open_input(&pFormatCtx, url.c_str(), nullptr, nullptr) != 0) {
cerr << "Failed to open input stream." << endl;
return -1;
}
if (avformat_find_stream_info(pFormatCtx, nullptr) < 0) {
cerr << "Failed to find stream information." << endl;
avformat_close_input(&pFormatCtx);
return -1;
}
for (int i = 0; i < pFormatCtx->nb_streams; i++) {
if (pFormatCtx->streams[i]->codecpar->codec_type == AVMEDIA_TYPE_VIDEO) {
videoStream = i;
break;
}
}
if (videoStream == -1) {
cerr << "Failed to find video stream." << endl;
avformat_close_input(&pFormatCtx);
return -1;
}
pCodec = avcodec_find_decoder(pFormatCtx->streams[videoStream]->codecpar->codec_id);
if (pCodec == nullptr) {
cerr << "Failed to find decoder." << endl;
avformat_close_input(&pFormatCtx);
return -1;
}
pCodecCtx = avcodec_alloc_context3(pCodec);
if (pCodecCtx == nullptr) {
cerr << "Failed to allocate codec context." << endl;
avformat_close_input(&pFormatCtx);
return -1;
}
if (avcodec_parameters_to_context(pCodecCtx, pFormatCtx->streams[videoStream]->codecpar) < 0) {
cerr << "Failed to copy codec parameters to context." << endl;
avcodec_free_context(&pCodecCtx);
avformat_close_input(&pFormatCtx);
return -1;
}
if (avcodec_open2(pCodecCtx, pCodec, nullptr) < 0) {
cerr << "Failed to open codec." << endl;
avcodec_free_context(&pCodecCtx);
avformat_close_input(&pFormatCtx);
return -1;
}
packet = av_packet_alloc();
if (packet == nullptr) {
cerr << "Failed to allocate packet." << endl;
avcodec_free_context(&pCodecCtx);
avformat_close_input(&pFormatCtx);
return -1;
}
AVFrame* pFrame = av_frame_alloc();
AVFrame* pFrameRGB = av_frame_alloc();
if (pFrame == nullptr || pFrameRGB == nullptr) {
cerr << "Failed to allocate frames." << endl;
av_packet_free(&packet);
avcodec_free_context(&pCodecCtx);
avformat_close_input(&pFormatCtx);
return -1;
}
int numBytes = av_image_get_buffer_size(AV_PIX_FMT_RGB24, pCodecCtx->width, pCodecCtx->height, 1);
uint8_t* buffer = (uint8_t*)av_malloc(numBytes * sizeof(uint8_t));
av_image_fill_arrays(pFrameRGB->data, pFrameRGB->linesize, buffer, AV_PIX_FMT_RGB24, pCodecCtx->width, pCodecCtx->height, 1);
struct SwsContext* swsCtx = sws_getContext(pCodecCtx->width, pCodecCtx->height, pCodecCtx->pix_fmt, pCodecCtx->width, pCodecCtx->height, AV_PIX_FMT_RGB24, SWS_BILINEAR, nullptr, nullptr, nullptr);
if (swsCtx == nullptr) {
cerr << "Failed to create sws context." << endl;
av_frame_free(&pFrame);
av_frame_free(&pFrameRGB);
av_packet_free(&packet);
avcodec_free_context(&pCodecCtx);
avformat_close_input(&pFormatCtx);
return -1;
}
while (av_read_frame(pFormatCtx, packet) >= 0) {
if (packet->stream_index == videoStream) {
if (avcodec_send_packet(pCodecCtx, packet) < 0) {
cerr << "Failed to send packet to decoder." << endl;
break;
}
while (avcodec_receive_frame(pCodecCtx, pFrame) >= 0) {
sws_scale(swsCtx, pFrame->data, pFrame->linesize, 0, pCodecCtx->height, pFrameRGB->data, pFrameRGB->linesize);
Mat img(pCodecCtx->height, pCodecCtx->width, CV_8UC3, pFrameRGB->data[0]);
imshow("RTSP Player", img);
waitKey(1);
av_frame_unref(pFrame);
}
}
av_packet_unref(packet);
}
sws_freeContext(swsCtx);
av_frame_free(&pFrame);
av_frame_free(&pFrameRGB);
av_packet_free(&packet);
avcodec_free_context(&pCodecCtx);
avformat_close_input(&pFormatCtx);
return 0;
}
```
请将`<your_rtsp_url>`替换为您要播放的RTSP URL。您还需要安装OpenCV库,因为该示例使用OpenCV显示视频帧。
希望这可以帮助你开始。
redis5.0.1
Redis是一个开源的内存数据库,用于存储数据、缓存和消息中间件。Redis 5.0.1是Redis的一个版本,它在Redis 5.0的基础上做了一些改进和优化。
Redis 5.0.1引入了Stream数据结构,这是一个新的数据类型,可以用来实现可持久化的消息队列。这个新特性使得Redis可以更好地支持消息传递和流处理,提高了Redis在实时应用和数据分析方面的能力。
另外,在Redis 5.0.1中,还加入了对TLS 1.3的支持,这意味着Redis可以在安全性能更强的TLS 1.3协议下进行加密通信,提高了数据传输的安全性。
此外,Redis 5.0.1还对命令的执行性能做了优化,提高了Redis在大规模数据处理时的效率和稳定性。
总的来说,Redis 5.0.1是Redis的一个新版本,它改进了消息传递和流处理的能力,提高了安全性能,并优化了命令执行性能。这些改进和优化使得Redis 5.0.1更适合于大规模数据处理和安全传输需求的场景。
阅读全文
相关推荐


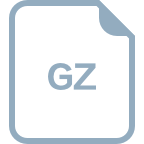










