React antd 实现拖拽组件
时间: 2023-11-20 13:07:26 浏览: 162
React Antd提供了一个Draggable组件,可以实现拖拽组件的功能。
首先,需要安装antd组件库和react-dnd库:
```bash
npm install antd react-dnd react-dnd-html5-backend
```
然后,在组件中引入Draggable组件和DragDropContext组件:
```jsx
import { Draggable } from 'react-drag-and-drop';
import { DragDropContext } from 'react-dnd';
import HTML5Backend from 'react-dnd-html5-backend';
import { Card } from 'antd';
const DragCard = ({ id, text, index, moveCard }) => {
const style = {
marginBottom: 16,
};
return (
<Draggable
key={id}
draggableId={id}
index={index}
onDragEnd={(result) => moveCard(result.source.index, result.destination?.index)}
>
{(provided) => (
<Card
{...provided.draggableProps}
{...provided.dragHandleProps}
ref={provided.innerRef}
style={{ ...provided.draggableProps.style, ...style }}
>
{text}
</Card>
)}
</Draggable>
);
};
const DragList = ({ cards, moveCard }) => {
return (
<div>
{cards.map((card, index) => (
<DragCard key={card.id} index={index} {...card} moveCard={moveCard} />
))}
</div>
);
};
const App = () => {
const [cards, setCards] = useState([
{ id: 'card-1', text: 'Card 1' },
{ id: 'card-2', text: 'Card 2' },
{ id: 'card-3', text: 'Card 3' },
]);
const moveCard = useCallback((sourceIndex, destinationIndex) => {
if (destinationIndex === undefined) {
return;
}
const newCards = [...cards];
const [removed] = newCards.splice(sourceIndex, 1);
newCards.splice(destinationIndex, 0, removed);
setCards(newCards);
}, [cards]);
return (
<DragDropContext backend={HTML5Backend}>
<DragList cards={cards} moveCard={moveCard} />
</DragDropContext>
);
};
```
在上述代码中,Draggable组件用于包裹需要拖拽的组件,而DragDropContext组件用于提供拖拽功能的上下文。通过onDragEnd回调函数,可以获取到拖拽的源位置和目标位置,然后更新数据源的位置。在拖拽的过程中,可以通过provided参数传递组件的属性,如样式等。
最后,将DragList组件渲染到页面中即可。
阅读全文
相关推荐
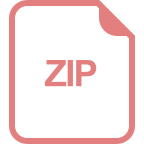
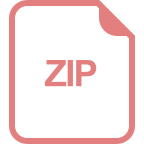



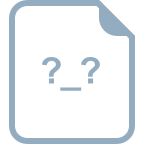
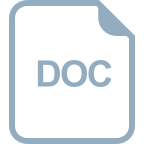

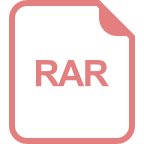
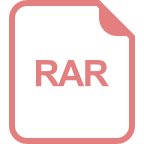
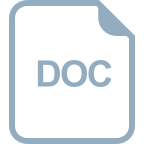


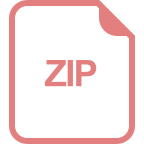
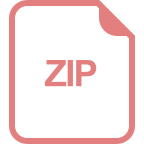
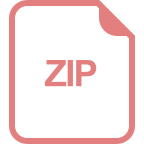

