写一个python脚本,在目标窗口输入自定义的内容
时间: 2023-08-31 11:36:33 浏览: 78
### 回答1:
你可以使用pyautogui库来实现这个功能。
```
import pyautogui
# 输入的内容
content = 'Hello, World!'
# 将鼠标移动到目标窗口并点击左键
pyautogui.moveTo(x, y)
pyautogui.click()
# 在目标窗口输入内容
pyautogui.typewrite(content)
```
需要注意的是,在上面的代码中,x和y是目标窗口的坐标,需要替换成你自己的坐标。
或者你也可以使用pywinauto库来自动化操作Windows窗口,代码类似如下:
```
from pywinauto.application import Application
app = Application().start("notepad.exe")
app.UntitledNotepad.Edit.SetText("Hello, World!")
```
这里我们让notepad程序输入内容,如果是其他程序,需要替换成相应程序名称。
### 回答2:
在Python中,我们可以使用第三方库pyautogui来模拟击键和鼠标操作来实现在目标窗口中输入自定义的内容。
首先,我们需要安装pyautogui库。使用pip命令可以很方便地进行安装:
```
pip install pyautogui
```
接下来,我们就可以开始编写Python脚本了。下面是一个简单的例子:
```python
import pyautogui
import time
def type_in_window(text):
# 延迟2秒钟,为了确保用户有足够的时间打开目标窗口
time.sleep(2)
# 获取目标窗口的位置
hwnd = pyautogui.getWindowsWithTitle('目标窗口的标题')[0].hwnd
rect = pyautogui.GetWindowRect(hwnd)
# 计算目标窗口的中心点
center_x = rect.left + (rect.right - rect.left) // 2
center_y = rect.top + (rect.bottom - rect.top) // 2
# 将鼠标移动到目标窗口的中心点,并点击一次确保窗口为活动窗口
pyautogui.moveTo(center_x, center_y)
pyautogui.click()
# 在目标窗口中输入指定的文本
pyautogui.typewrite(text)
# 调用函数,输入自定义的内容
type_in_window("Hello, World!")
```
在上述代码中,我们首先使用`time.sleep(2)`延迟2秒钟,等待用户打开目标窗口。然后,通过`pyautogui.getWindowsWithTitle('目标窗口的标题')[0].hwnd`获取目标窗口的句柄,并使用`pyautogui.GetWindowRect(hwnd)`获取目标窗口的位置。接着,计算目标窗口的中心点,通过`pyautogui.moveTo(center_x, center_y)`将鼠标移动到目标窗口的中心点,并使用`pyautogui.click()`点击一次,确保目标窗口为活动窗口。最后,使用`pyautogui.typewrite(text)`在目标窗口中输入指定的文本。
请注意,上述代码中的`'目标窗口的标题'`需要替换为实际目标窗口的标题。在使用pyautogui的时候,还要根据实际需求,进行一些适当的调整和优化。
### 回答3:
要实现在目标窗口输入自定义的内容,可以借助`pyautogui`库来完成。下面是一个示例的Python脚本:
```python
import pyautogui
import time
def input_content(target_window, content):
# 等待目标窗口出现
while True:
try:
target_pos = pyautogui.locateOnScreen(target_window)
if target_pos is not None:
break
except Exception as e:
print(e)
time.sleep(1)
# 移动鼠标到目标窗口位置并点击
target_center = pyautogui.center(target_pos)
pyautogui.click(target_center)
# 输入内容
pyautogui.typewrite(content)
# 模拟按下回车键
pyautogui.press('enter')
# 在目标窗口输入内容
input_content('target_window.png', '自定义内容')
```
以上脚本首先使用`pyautogui.locateOnScreen()`函数来定位目标窗口的位置,该函数需要传入目标窗口的截图路径(可以使用`pyautogui.screenshot()`函数先截取目标窗口的截图),返回目标窗口在屏幕上的位置。然后使用`pyautogui.center()`函数获取目标窗口位置的中心点坐标,并使用`pyautogui.click()`函数移动鼠标到目标窗口位置并点击。接下来使用`pyautogui.typewrite()`函数输入自定义的内容,最后使用`pyautogui.press()`函数模拟按下回车键。最终实现在目标窗口输入自定义的内容。
相关推荐
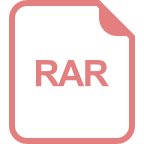
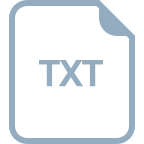
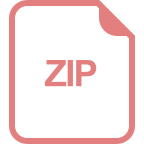














