double free detected in tcache
时间: 2023-04-18 11:00:09 浏览: 101
这是一个内存错误,通常在使用C或C++编程语言时出现。它表示尝试释放已经被释放的内存,这通常会导致程序崩溃或未定义的行为。
这种错误通常是由于两个或多个函数尝试释放同一个内存地址,或者在释放内存后仍然尝试访问该内存地址而引起的。解决此错误的方法通常是仔细检查代码并确保内存被正确分配和释放。可以使用内存分配和释放函数(例如malloc和free)来帮助管理内存。
如果这个问题在使用第三方库时出现,那么可能需要查看库的文档或寻求支持来解决这个问题。
相关问题
运行上面的代码出现buf= free(): double free detected in tcache 2
这个错误通常是因为重复释放了同一个指针所导致的。在你的代码中,出现这个错误的可能原因是:在调用 `replaceChar()` 函数之后,由于你在函数中使用了 `realloc()` 函数重新分配了 `str` 指向的内存空间,而在 `main()` 函数中,你使用 `free()` 函数释放了 `buf` 指向的内存空间,这就导致了重复释放同一个指针的错误。
解决这个问题的方法是:在 `replaceChar()` 函数中不要直接修改 `str` 指针的值,而是应该返回一个新的指针,指向重新分配的内存空间。在 `main()` 函数中,你就可以释放这个新的指针所指向的内存空间,而不会出现重复释放的问题。修改后的代码示例如下:
```
char* replaceChar(char* str, char chr, char* new_str) {
int old_len = strlen(str);
int new_len = strlen(new_str);
int count = 0;
char* p = str;
while (*p != '\0') {
if (*p == chr) {
count++;
}
p++;
}
if (count == 0) {
return str;
}
int new_size = old_len + count * (new_len - 1);
char* new_str_ptr = (char*)realloc(str, new_size + 1);
if (new_str_ptr == NULL) {
return str;
}
str = new_str_ptr;
char* dst = str + old_len + count * (new_len - 1);
char* src = str + old_len;
while (src >= str) {
if (*src == chr) {
memcpy(dst - new_len + 1, new_str, new_len);
dst -= new_len;
} else {
*dst = *src;
dst--;
}
src--;
}
return str;
}
int main() {
char* buf = strdup("hello123456");
printf("buf=%s\n", buf);
buf = replaceChar(buf, 'o', "aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa");
printf("buf=%s\n", buf);
free(buf);
return 0;
}
```
在修改后的代码中,`replaceChar()` 函数返回一个新的指针 `new_str_ptr`,指向重新分配的内存空间,而不是直接修改原来的指针 `str` 的值。在 `main()` 函数中,你使用新的指针 `buf` 来指向 `replaceChar()` 函数返回的内存空间,这样就可以避免重复释放同一个指针的错误。
divergence detected in amg solver: k
"divergence detected in amg solver: k" 是一个关于 AMG (代数多重网格) 求解器中发现分歧的错误信息。
AMG 是一种用于求解线性系统的求解器,特别适用于稀疏矩阵和大规模问题。在求解器的迭代过程中,可能会出现分歧现象,这意味着求解器无法继续进行下去并找到解。
出现这种错误信息可能有多种原因,包括初始猜测的不准确性、问题的特殊属性或模型等。在这种情况下,我们可以考虑一些方法来解决这个问题:
1. 检查线性系统的输入:确保正确地设置了问题的边界条件、初始条件和系数矩阵。验证输入是否准确无误。
2. 检查收敛准则:检查是否设置了适当的迭代终止准则,例如残差或误差限。
3. 检查矩阵的性质:分析问题的特殊属性或模型,特别是可能导致分歧的部分。尝试使用其他的求解器或预处理器。
4. 修改求解器参数:尝试调整求解器的参数,如迭代步数、松弛因子等。
如果上述方法仍然无法解决问题,我们可能需要进一步研究和分析问题的特点,或者考虑使用其他求解器或方法来解决分歧问题。
相关推荐
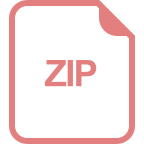
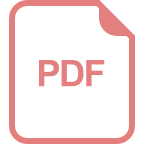
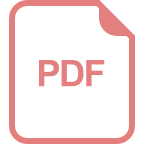












