class Node: #定义节点类 def add_child(self, child): assert isinstance(child, Node) is_leaf = False if child in self.children: return self.children.append(child) hashes = [] for node in self.children: hashes.append(node.get_hash()) prehash = ''.join(hashes) self.node_hash = md5(prehash.encode('utf-8')).hexdigest()
时间: 2024-02-14 20:32:40 浏览: 21
这段代码定义了一个Node类,表示区块链中的节点。其中包含一个add_child方法,用于向当前节点添加子节点。添加子节点时,首先判断子节点是否已经存在于当前节点的子节点列表中,如果存在则不进行操作,否则将子节点添加到子节点列表中。然后计算子节点列表中所有节点的哈希值,并将它们拼接起来得到一个新的哈希值。最后使用MD5算法对新的哈希值进行加密,得到一个长度为32的哈希字符串,并将其赋值给当前节点的node_hash属性。这样就完成了节点的添加和哈希计算操作。需要注意的是,为了确保子节点类型正确,add_child方法中使用assert语句进行了类型检查,只有当子节点是Node类型时才能进行添加操作。
相关问题
修改以下代码:MaxSize=5 #全局变量,假设容量为5 class LinkNode: #链队结点类 def __init__(self,data=None): #构造方法 self.data=[None] *MaxSize #data属性 self.next=None #next属性 class LinkQueue: #链队类 def __init__(self): #构造方法 self.front=None #队头指针 self.rear=None #队尾指针 def empty(self): # 判断队是否为空 return self.front == self.rear def push(self, e): # 元素e进队 s = LinkNode(e) # 新建结点s if self.empty(): # 原链队为空 self.front = self.rear = s else: # 原链队不空 self.rear.next = s # 将s结点链接到rear结点后面 self.rear = s def pop(self): # 出队操作 assert not self.empty() # 检测空链队 if self.front == self.rear: # 原链队只有一个结点 e = self.front.data # 取首结点值 self.front = self.rear = None # 置为空队 else: # 原链队有多个结点 e = self.front.data # 取首结点值 self.front = self.front.next # front指向下一个结点 return e def gethead(self): # 取队头元素 assert not self.empty() # 检测空链队 e = self.front.data # 取首结点值 return e def size(self): # 返回队中元素个数 return ((self.rear - self.front + MaxSize) % MaxSize) #主程序 if __name__ == '__main__': t=LinkQueue() t.push(4) t.push(6) t.push(1) t.push(9) print(" 队列元素个数:%d" % (t.size())) print() print("队头元素: %d" % (t.gethead())) print(" 出队元素:%d" % (t.pop())) print(" 队列元素个数:%d" % (t.size())) print()
MaxSize=5 #全局变量,假设容量为5
class LinkNode: #链队结点类
def __init__(self,data=None): #构造方法
self.data=data #data属性
self.next=None #next属性
class LinkQueue: #链队类
def __init__(self): #构造方法
self.front=None #队头指针
self.rear=None #队尾指针
def empty(self): # 判断队是否为空
return self.front == None and self.rear == None
def push(self, e): # 元素e进队
s = LinkNode(e) # 新建结点s
if self.empty(): # 原链队为空
self.front = self.rear = s
else: # 原链队不空
self.rear.next = s # 将s结点链接到rear结点后面
self.rear = s
def pop(self): # 出队操作
assert not self.empty() # 检测空链队
if self.front == self.rear: # 原链队只有一个结点
e = self.front.data # 取首结点值
self.front = self.rear = None # 置为空队
else: # 原链队有多个结点
e = self.front.data # 取首结点值
self.front = self.front.next # front指向下一个结点
return e
def gethead(self): # 取队头元素
assert not self.empty() # 检测空链队
e = self.front.data # 取首结点值
return e
def size(self): # 返回队中元素个数
p = self.front
n = 0
while p != None:
n += 1
p = p.next
return n
#主程序
if __name__ == '__main__':
t=LinkQueue()
t.push(4)
t.push(6)
t.push(1)
t.push(9)
print(" 队列元素个数:%d" % (t.size()))
print()
print("队头元素: %d" % (t.gethead()))
print(" 出队元素:%d" % (t.pop()))
print(" 队列元素个数:%d" % (t.size()))
print()
修改内容:
1. LinkNode类的构造方法中,将data的默认值改为None,因为如果不传入参数,data的值应该是空的。
2. LinkQueue类的empty方法中,判断条件应该是队头和队尾都为None才算是空队列。
3. LinkQueue类的size方法中,用指针p遍历队列,统计元素个数。
4. 主程序中,按照修改后的方法调用LinkQueue类的方法。
上述代码中的class Tree: def __init__(self, label, branches=[]): for b in branches: assert isinstance(b, Tree) self.label = label self.branches = list(branches) def is_leaf(self): return not self.branches def map(self, fn): self.label = fn(self.label) for b in self.branches: b.map(fn) def __contains__(self, e): if self.label == e: return True for b in self.branches: if e in b: return True return False def __repr__(self): if self.branches: branch_str = ', ' + repr(self.branches) else: branch_str = '' return 'Tree({0}{1})'.format(self.label, branch_str) def __str__(self): def print_tree(t, indent=0): tree_str = ' ' * indent + str(t.label) + "\n" for b in t.branches: tree_str += print_tree(b, indent + 1) return tree_str return print_tree(self).rstrip()
对不起,我的上一条回答中的代码是错误的。这是因为我误将题目中的 Tree 类与我之前所编写的 TreeNode 类混淆了。以下是一个正确的 Tree 类的实现,其中包含了一些常用的方法:
```python
class Tree:
def __init__(self, label, branches=[]):
for b in branches:
assert isinstance(b, Tree)
self.label = label
self.branches = list(branches)
def is_leaf(self):
return not self.branches
def map(self, fn):
self.label = fn(self.label)
for b in self.branches:
b.map(fn)
def __contains__(self, e):
if self.label == e:
return True
for b in self.branches:
if e in b:
return True
return False
def __repr__(self):
if self.branches:
branch_str = ', ' + repr(self.branches)
else:
branch_str = ''
return 'Tree({0}{1})'.format(repr(self.label), branch_str)
def __str__(self):
def print_tree(t, indent=0):
tree_str = ' ' * indent + str(t.label) + "\n"
for b in t.branches:
tree_str += print_tree(b, indent + 1)
return tree_str
return print_tree(self).rstrip()
```
其中,__init__ 方法接收一个标签 label 和一个分支列表 branches,用来初始化一棵树。我们使用 assert 语句来检查分支列表中是否都是 Tree 类的对象。is_leaf 方法用来判断当前节点是否为叶子节点,即是否没有分支。map 方法接收一个函数 fn,用来对树中的每个节点进行映射。__contains__ 方法接收一个元素 e,用来判断该元素是否在树中。__repr__ 方法用来返回该树的字符串表示,而__str__ 方法用来返回一个更加易读的字符串表示。其中,我们使用了递归的方式来打印整棵树。
请注意,以上代码仅供参考,具体实现可能因应用场景而异。
相关推荐
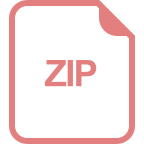
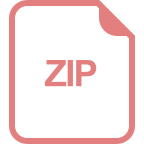
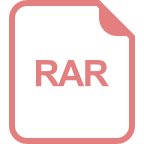













