android应用下载安装apk升级版本实现demo适配Android30
时间: 2024-09-06 20:08:22 浏览: 45
在Android应用开发中,下载并安装APK以升级应用版本通常涉及几个步骤:下载APK文件、安装APK文件以及确保新版本与Android 30的兼容性。以下是一个简化的过程和示例代码来说明如何实现这样的升级机制。
1. **下载APK文件**:首先,你需要从服务器下载新版本的APK文件。这通常通过网络请求来实现,比如使用OkHttp库下载文件。
```java
// 示例代码,用于从网络下载APK文件
OkHttpClient client = new OkHttpClient();
String url = "http://yourserver.com/yourapp.apk"; // 替换为实际的APK下载链接
Request request = new Request.Builder()
.url(url)
.build();
client.newCall(request).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
// 处理下载失败的情况
}
@Override
public void onResponse(Call call, Response response) throws IOException {
if (response.isSuccessful()) {
// 下载成功,保存APK文件到应用的私有目录
File apkFile = new File(getFilesDir(), "update.apk");
try (FileOutputStream fos = new FileOutputStream(apkFile);
InputStream is = response.body().byteStream()) {
byte[] buffer = new byte[1024];
int len;
while ((len = is.read(buffer)) != -1) {
fos.write(buffer, 0, len);
}
}
// 下载完成后,调用安装APK的方法
installApk(apkFile);
}
}
});
```
2. **安装APK文件**:一旦APK文件下载并保存在设备上,你可以使用`Intent`和`Uri`来启动安装过程。注意,从Android N(7.0)开始,需要适配“FileProvider”来分享文件。
```java
// 示例代码,用于安装APK文件
private void installApk(File apkFile) {
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.N) {
Uri apkUri = FileProvider.getUriForFile(this, getPackageName() + ".provider", apkFile);
Intent intent = new Intent(Intent.ACTION_VIEW);
intent.setFlags(Intent.FLAG_ACTIVITY_NEW_TASK);
intent.setDataAndType(apkUri, "application/vnd.android.package-archive");
startActivity(intent);
} else {
Intent intent = new Intent(Intent.ACTION_VIEW);
intent.setDataAndType(Uri.fromFile(apkFile), "application/vnd.android.package-archive");
intent.setFlags(Intent.FLAG_ACTIVITY_NEW_TASK);
startActivity(intent);
}
}
```
3. **适配Android 30**:为了确保新版本应用与Android 30的兼容性,你需要遵循新的权限模型、存储访问框架(Scoped Storage)以及隐私相关的更新。例如,更新你的应用以使用分区存储。
```xml
<!-- 在AndroidManifest.xml中添加属性以启用分区存储 -->
<manifest ...>
<application android:requestLegacyExternalStorage="true">
...
</application>
</manifest>
```
请注意,`requestLegacyExternalStorage`是一个过渡属性,用于在Android 10上请求完整的外部存储访问权限。对于Android 11及以上版本,你可能需要使用分区存储或者动态请求权限。
相关推荐
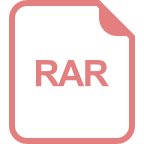
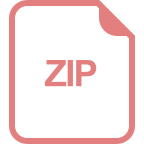
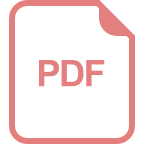














