OLED_ShowString(2,0,"Hongwai:",9);报错warning:passing 'char[9]'to parameter of type'unsigned char *'converts between point
时间: 2024-02-02 16:05:34 浏览: 86
这个问题可能是因为函数`OLED_ShowString`的第三个参数需要一个`unsigned char *`类型的指针,而你传递了一个`char[9]`类型的数组。
要解决这个问题,你可以尝试将数组转换为指针,或者将数组声明为`unsigned char`类型。
以下是两种可能的解决方法:
方法1:将数组转换为指针
```cpp
char text[] = "Hongwai:";
OLED_ShowString(2, 0, (unsigned char *)text, 9);
```
方法2:将数组声明为`unsigned char`类型
```cpp
unsigned char text[] = "Hongwai:";
OLED_ShowString(2, 0, text, 9);
```
通过以上两种方法,你应该能够解决这个警告问题。记得在实际使用时确认参数的正确性以及其他相关代码的正确性。
相关问题
incompatible integer to pointer conversion passing u8' (aka 'unsigned char') to parameter of type 'char *
这个错误提示意味着您正在尝试将一个无符号字符(unsigned char)传递给一个字符指针(char *)类型的参数,这两种类型在C/C++中是不兼容的。
在C/C++中,无符号字符(unsigned char)和字符(char)虽然在值上没有区别,但它们在类型上是不同的。当您将一个无符号字符传递给一个字符指针参数时,编译器会发出警告或错误,因为它们的类型不匹配。
要解决这个问题,您可以考虑以下几种方法:
1. 将无符号字符转换为字符类型:您可以使用强制类型转换将无符号字符转换为字符类型,即将unsigned char类型的值转换为char类型的值。例如:`(char)u8'`
2. 修改参数类型:如果函数或方法的参数类型允许接收无符号字符类型,您可以将参数的类型更改为unsigned char *或const unsigned char *,以便接受无符号字符指针。
请记住,在进行类型转换时,要确保您对数据的含义和范围有正确的理解,并确保转换是安全的。如果可能的话,尽量避免进行不必要的类型转换,以减少潜在的错误和问题。
mb_detect_encoding(): passing null to parameter
在使用 `mb_detect_encoding()` 函数时,如果传递了一个空字符串或者变量,会导致出现 `mb_detect_encoding(): passing null to parameter` 的错误提示。这是因为该函数要求第一个参数必须是一个非空字符串。
为了避免这个错误,我们可以在调用该函数之前,先使用 `isset()` 或 `empty()` 函数来判断字符串是否为空。如果字符串为空,可以给它一个默认值或者直接返回错误提示。
以下是一个示例代码:
```
$str = ""; // 空字符串
if (isset($str) && !empty($str)) {
$encoding = mb_detect_encoding($str, "ASCII,UTF-8,GB2312,GBK,BIG5");
echo $encoding;
} else {
echo "字符串为空";
}
```
在上述代码中,我们先使用 `isset()` 和 `empty()` 函数来判断字符串是否为空。如果字符串不为空,就调用 `mb_detect_encoding()` 函数来检测编码类型。如果字符串为空,就直接输出错误提示。这样就可以避免出现 `mb_detect_encoding(): passing null to parameter` 的错误提示了。
相关推荐
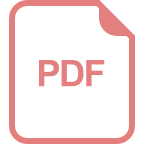
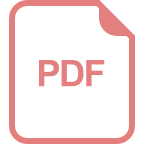
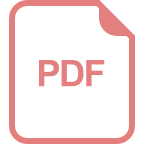













