AttributeError: 'list' object has no attribute 'move'. Did you mean: 'remove'?
时间: 2024-08-09 22:01:38 浏览: 67
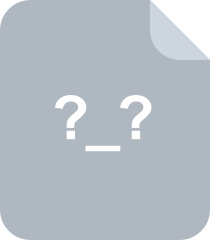
AttributeError: module 'tensorflow.compat.v1' has no attribute '
当你在Python程序中遇到`AttributeError: 'list' object has no attribute 'move'`这个错误信息时,通常意味着你在尝试访问或操作一个列表时使用的属性名或方法名是错误的。在这种情况下,提示中提到“Did you mean: 'remove’?”意味着可能是你想删除列表中的某个元素,却误用了 `move` 方法。
### 列表的基本操作
Python 中列表是一种有序的数据集合,可以存储多种数据类型的元素。常用的列表操作包括:
#### 添加元素
- 使用 `append()` 将元素添加到列表末尾。
- 使用 `extend()` 合并两个列表。
- 使用 `insert(index, element)` 将元素插入到指定位置。
#### 删除元素
- 使用 `remove(element)` 移除第一个匹配指定值的元素。
- 使用 `pop([index])` 删除并返回列表的最后一个元素,默认情况下,如果没有提供索引,则会删除并返回最后一个元素;如果提供了索引,则删除该索引处的元素,并返回其值。
- 使用 `del list[index]` 直接从列表中删除指定索引处的元素。
#### 查找元素
- 使用 `index(element[, start[, end]])` 返回指定元素的第一个匹配项的索引位置。如果未找到该元素,将引发 `ValueError`。
#### 修改元素
- 可以直接通过索引来修改列表中的元素值,例如 `my_list = new_value`。
### 错误示例解析
如果你试图使用 `move` 来操作列表而得到上述错误,那么很可能是因为你尝试了列表不支持的操作 `move`。实际上,对于列表来说,正确的删除操作是使用 `remove` 或 `pop` 函数之一。
### 解决方案
假设你想要删除列表中的某个特定元素,比如名称为 `name` 的元素,应该使用下面的方式:
```python
# 示例列表
names = ['Alice', 'Bob', 'Charlie']
# 想要删除 'Bob'
target_name = 'Bob'
# 使用 remove 方法
if target_name in names:
names.remove(target_name)
else:
print(f"{target_name} not found in the list.")
print("Updated list:", names)
```
或者如果你想按照索引删除元素:
```python
# 示例列表及目标索引
names = ['Alice', 'Bob', 'Charlie']
index_to_remove = 1
# 使用 pop 方法
try:
removed_item = names.pop(index_to_remove)
print(f"Removed item: {removed_item}")
except IndexError:
print(f"Index {index_to_remove} out of range.")
print("Updated list:", names)
```
###
阅读全文
相关推荐
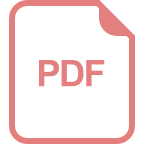
















