用C语言编写一个算法函数,使用链栈或队列,判定给定的字符序列是否为回文,并验证。 提示:回文是指正读和反读均相同的字符序列。要有输入。不能用strlen函数。
时间: 2024-10-16 12:05:09 浏览: 40
在C语言中,我们可以使用链表作为数据结构来实现栈和队列的功能,用于判断一个字符串是否是回文。这里我将演示如何使用链表栈来实现这个功能,因为链表栈更适合做双端访问。
首先,我们需要创建链表节点结构以及链表相关的头文件和函数:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构
typedef struct Node {
char data;
struct Node* next;
} Node;
// 创建新节点
Node* createNode(char data) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (!newNode) {
printf("Memory allocation failed.\n");
return NULL;
}
newNode->data = data;
newNode->next = NULL;
return newNode;
}
// 添加元素到链表栈顶
void push(Node** top, char data) {
Node* newNode = createNode(data);
if (newNode) {
newNode->next = *top;
*top = newNode;
} else {
printf("Failed to allocate memory for new node.\n");
}
}
// 检查链表是否为空
int isEmpty(Node* top) {
return top == NULL;
}
// 比较两个链表是否相等,用于判断回文
int compareLists(Node* list1, Node* list2) {
while (list1 && list2) {
if (list1->data != list2->data) {
return 0; // 如果有不同,不是回文
}
list1 = list1->next;
list2 = list2->next;
}
if (list1 || list2) {
return 0; // 如果有一个到达末尾而另一个没到,不是回文
}
return 1; // 如果都到了末尾,是回文
}
// 主函数用于检测输入字符串是否为回文
int isPalindrome(char str[]) {
int len = 0;
Node* stackTop = NULL;
Node* tempStack = NULL;
// 遍历字符串并将每个字符推入栈
for (int i = 0; str[i]; ++i) {
push(&stackTop, str[i]);
len++;
}
// 清理并反转栈,同时比较原顺序和翻转后的顺序
tempStack = stackTop;
while (!isEmpty(stackTop)) {
if (compareLists(tempStack, stackTop) == 0) {
tempStack = tempStack->next;
stackTop = stackTop->next;
} else {
return 0; // 不是回文
}
}
return 1; // 是回文
}
// 测试函数
int main() {
char str[] = "radar";
if (isPalindrome(str)) {
printf("%s is a palindrome.\n", str);
} else {
printf("%s is not a palindrome.\n", str);
}
return 0;
}
```
在这个程序中,`isPalindrome`函数首先将输入字符串中的字符逐个压入链表栈,然后清理并反转栈,最后对比原顺序和反转顺序的链表内容,如果两者相等,则该字符串是回文。
阅读全文
相关推荐
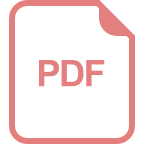
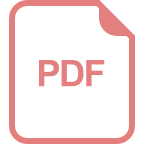
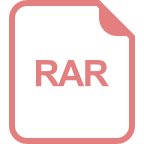















