buttonClick
时间: 2024-08-12 18:09:46 浏览: 136
"buttonClick"通常是一个函数名,它可能用于处理用户点击按钮的事件。在编程中,这个函数可能会执行一些操作,比如导航到新的页面、触发数据提交、执行某个逻辑处理等,具体取决于它被设计的上下文和功能。按钮(Button)是用户界面中的常见元素,其click事件是用户交互的关键部分。
举个例子,在JavaScript中,你可能会这样定义一个buttonClick函数:
```javascript
function buttonClick(event) {
event.preventDefault(); // 阻止默认行为(如表单提交)
alert('Button clicked!'); // 显示警告消息
// 或者更复杂的操作,如导航或调用API
}
```
相关问题
wpf button使用Binding绑定Click事件
在 WPF 中,可以使用 Binding 绑定 Click 事件,但需要使用一个特殊的附加属性来实现。具体步骤如下:
1. 在 XAML 中定义 Button 控件,并使用 Binding 绑定 Click 事件,例如:
```
<Button Content="Click Me" local:ButtonClick.Command="{Binding MyCommand}" />
```
其中,ButtonClick 是一个自定义附加属性,可以在代码中定义,MyCommand 是一个 ICommand 类型的属性,表示要执行的命令。
2. 在代码中定义 ButtonClick 附加属性,并在其 PropertyChangedCallback 中注册 Click 事件的处理方法,例如:
```
public static class ButtonClick
{
public static readonly DependencyProperty CommandProperty =
DependencyProperty.RegisterAttached("Command", typeof(ICommand), typeof(ButtonClick),
new FrameworkPropertyMetadata(null, CommandChanged));
public static ICommand GetCommand(Button button)
{
return (ICommand)button.GetValue(CommandProperty);
}
public static void SetCommand(Button button, ICommand value)
{
button.SetValue(CommandProperty, value);
}
private static void CommandChanged(DependencyObject d, DependencyPropertyChangedEventArgs e)
{
if (d is Button button)
{
button.Click -= Button_Click;
if (e.NewValue is ICommand command)
{
button.Click += Button_Click;
}
}
}
private static void Button_Click(object sender, RoutedEventArgs e)
{
if (sender is Button button)
{
ICommand command = GetCommand(button);
if (command != null && command.CanExecute(button.CommandParameter))
{
command.Execute(button.CommandParameter);
}
}
}
}
```
其中,CommandProperty 是 ButtonClick 附加属性的依赖属性,GetCommand 和 SetCommand 方法用于获取和设置 Command 属性的值,CommandChanged 方法在 Command 属性值发生变化时注册或取消注册 Click 事件的处理方法,Button_Click 方法是 Click 事件的处理方法,用于执行绑定的命令。
注意,使用这种方式绑定 Click 事件时,Button 控件的 Click 事件不会触发,而是会触发 ButtonClick 附加属性的处理方法。
this.button1.Click += new System.EventHandler(this.button1_Click);
这是一个 C# 代码片段,它为按钮添加了一个单击事件处理程序。当用户单击按钮时,该事件处理程序将被触发。具体来说,代码中的 this.button1 表示窗体中名为 button1 的按钮控件,Click 表示单击事件,new System.EventHandler(this.button1_Click) 表示新建一个事件处理程序对象并将其绑定到 button1 的 Click 事件上,this.button1_Click 则是事件处理程序的方法名。
阅读全文
相关推荐
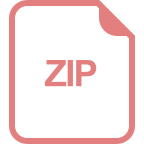
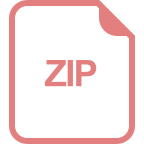
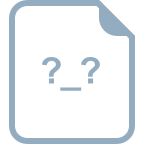
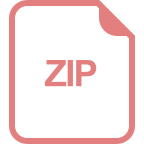
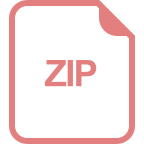
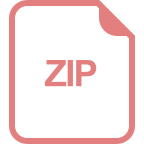
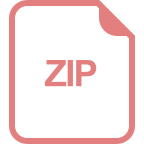
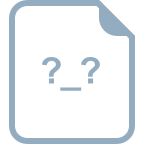
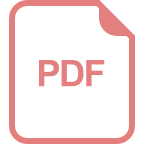
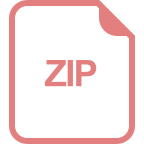
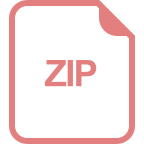
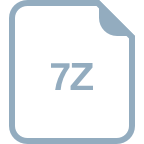
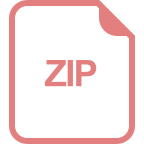
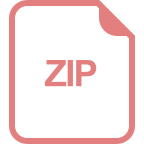
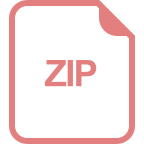
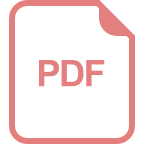
