用codeblocks 输入10个同学的座号,2门课成绩,按总分由高到低排序,并输出。
时间: 2024-11-20 20:40:17 浏览: 9
这是一个简单的C++程序示例,使用Code::Blocks编写,假设我们有一个学生结构体(Student),包含座号、两门课的成绩(score1和score2),然后计算总分并按照总分从高到低排序:
```cpp
#include <iostream>
#include <vector>
#include <algorithm> // 包含sort算法
// 定义学生结构体
struct Student {
int id; // 座号
int score1; // 第一门课成绩
int score2; // 第二门课成绩
};
// 比较函数用于排序
bool compareTotal(const Student& a, const Student& b) {
return (a.score1 + a.score2) > (b.score1 + b.score2);
}
int main() {
std::vector<Student> students;
// 输入10个同学的信息
for (int i = 0; i < 10; ++i) {
Student s;
std::cout << "请输入第" << i+1 << "位同学的座号及两门课成绩:\n";
std::cin >> s.id >> s.score1 >> s.score2;
students.push_back(s);
}
// 按照总分排序
std::sort(students.begin(), students.end(), compareTotal);
// 输出结果
std::cout << "按总分从高到低排列的结果:" << std::endl;
for (const auto& student : students) {
std::cout << "座号: " << student.id << ", 总分: " << (student.score1 + student.score2) << std::endl;
}
return 0;
}
```
阅读全文
相关推荐
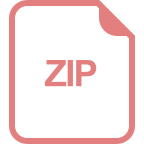
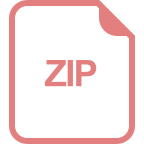
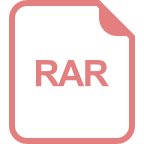
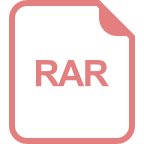








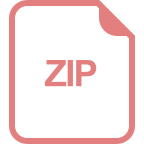
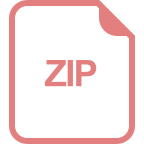
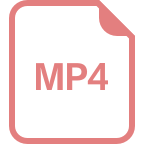