C# 获取http路径文件多少kb到多少kb 并且转为数据流Blob格式 不要异步请求
时间: 2024-10-15 20:15:20 浏览: 23
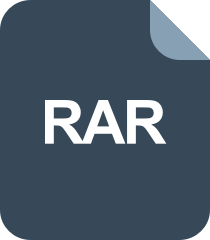
.net通过文件流获取对方请求过来的文件

在C#中,获取HTTP路径文件并将其转换为指定大小的数据流(Blob)格式,可以使用`HttpClient`和`FileStream`来完成同步操作。以下是一个简单的示例,假设我们有一个URL `url`,我们需要下载的范围是从`startKB`到`endKB`:
```csharp
using System;
using System.IO;
using System.Net.Http;
class Program
{
static async Task Main(string[] args)
{
string url = "http://yourfile.com"; // 替换为实际的URL
int startKB = 10; // 开始下载的位置(以KB为单位)
int endKB = 50; // 结束下载的位置(以KB为单位)
byte[] chunk = await DownloadRange(url, startKB * 1024, endKB * 1024);
if (chunk != null)
{
Stream blobStream = new MemoryStream(chunk);
// 假设blobStream就是你的Blob数据流
}
}
private static async Task<byte[]> DownloadRange(string url, long startByte, long endByte)
{
HttpClient httpClient = new HttpClient();
HttpResponseMessage response = await httpClient.GetAsync(url);
if (!response.IsSuccessStatusCode)
{
Console.WriteLine($"Error: {response.StatusCode}");
return null;
}
using (HttpResponseContent content = response.Content)
{
HttpContentHeaders headers = content.Headers;
long contentLength = headers.ContentLength.Value;
if (contentLength == -1 || startByte >= contentLength || endByte > contentLength)
{
Console.WriteLine("Invalid range or file size is unknown.");
return null;
}
Stream contentStream = await content.ReadAsStreamAsync();
byte[] buffer = new byte[1024];
using (MemoryStream rangeStream = new MemoryStream())
{
int bytesRead;
while ((bytesRead = contentStream.Read(buffer, 0, buffer.Length)) > 0)
{
long position = contentStream.Position;
if (position < startByte)
{
continue;
}
if (position >= endByte * 1024)
{
break;
}
rangeStream.Write(buffer, 0, bytesRead);
}
return rangeStream.ToArray();
}
}
}
}
```
这个示例首先发送一个GET请求到指定的URL,然后检查响应状态码是否成功。如果成功,它读取内容并在范围内写入到新的内存流中。如果没有达到指定的范围,它只返回已知范围内的数据。
注意,这只是一个基础示例,实际应用中可能需要处理更多的异常情况和错误。另外,如果你确实想要创建一个Blob,你可能需要使用Azure存储库或其他云存储服务提供的API,这在此示例中没有直接体现,但大体逻辑类似。
阅读全文
相关推荐
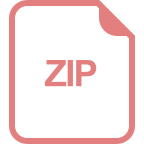
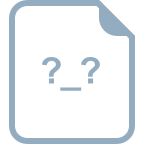
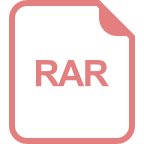
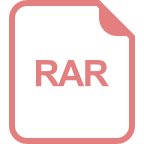
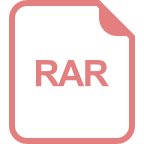
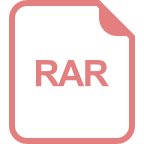
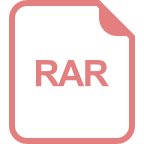
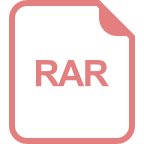
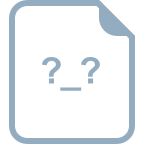
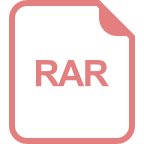
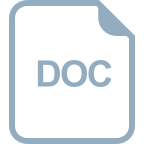
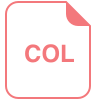
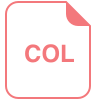
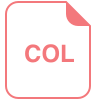
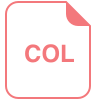
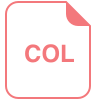
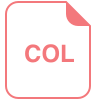