c语言通讯录管理系统代码
时间: 2024-11-20 08:26:01 浏览: 88
C语言编写一个简单的通讯录管理系统,我们可以创建一个结构体来存储联系人信息,然后提供添加、删除、搜索等功能。以下是一个基本的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义联系人结构体
typedef struct Contact {
char name[50];
char phone[20];
} Contact;
// 创建联系人列表
Contact* create_contact_list() {
Contact* contacts = malloc(sizeof(Contact));
contacts->name = "";
contacts->phone = "";
return contacts;
}
// 添加联系人
void add_contact(Contact** list, char* name, char* phone) {
Contact* new_contact = malloc(sizeof(Contact));
strcpy(new_contact->name, name);
strcpy(new_contact->phone, phone);
*list = realloc(*list, sizeof(Contact) + sizeof(Contact*) * (*list ? 1 : 0));
(*list)[(*list)->size++] = new_contact;
}
// 搜索联系人
Contact* search_contact(Contact* list, char* name) {
for (int i = 0; i < list->size; i++) {
if (strcmp(list->data[i].name, name) == 0) {
return &list->data[i];
}
}
return NULL;
}
// 删除联系人
void delete_contact(Contact** list, char* name) {
for (int i = 0; i < list->size; i++) {
if (strcmp(list->data[i].name, name) == 0) {
free(list->data[i]);
memmove(&list->data[i], &list->data[i+1], (list->size - i - 1) * sizeof(Contact));
list->size--;
break;
}
}
}
// 清理内存
void destroy_contact_list(Contact** list) {
for (int i = 0; i < (*list)->size; i++) {
free((*list)->data[i]);
}
free(*list);
*list = NULL;
}
int main() {
Contact* contact_list = create_contact_list();
// 添加联系人...
add_contact(&contact_list, "张三", "13812345678");
// ...其他操作...
// 搜索联系人
Contact* found = search_contact(contact_list, "张三");
if (found) {
printf("Found contact: %s, Phone: %s\n", found->name, found->phone);
} else {
printf("Contact not found.\n");
}
// 删除联系人
delete_contact(&contact_list, "张三");
destroy_contact_list(&contact_list);
return 0;
}
```
注意,这个示例没有处理错误情况,实际应用中需要完善错误检查。此外,你可以根据需求增加更多的功能,如遍历显示整个联系人列表等。
阅读全文
相关推荐










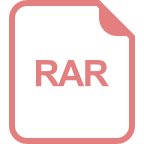




