yolov5行人检测界面
时间: 2023-08-01 08:12:28 浏览: 51
你好!以下是一个基于YOLOv5的行人检测界面的示例代码:
```python
import cv2
import torch
from PIL import Image
from torchvision.transforms import functional as F
# 加载模型
model = torch.hub.load('ultralytics/yolov5', 'yolov5s', pretrained=True).autoshape()
# 设置设备
device = torch.device('cuda' if torch.cuda.is_available() else 'cpu')
model = model.to(device).eval()
# 定义类别标签
classes = ['person']
def detect_pedestrians(image_path):
# 加载图像
image = Image.open(image_path)
# 图像预处理
image = F.to_tensor(image).unsqueeze(0)
image = image.to(device)
# 进行行人检测
results = model(image)
# 解析检测结果
boxes = results.xyxy[0] # 边界框坐标
confidences = boxes[:, 4] # 置信度分数
# 绘制边界框和标签
for box, confidence in zip(boxes, confidences):
if confidence > 0.5:
x1, y1, x2, y2 = box[:4].tolist()
label = f'{classes[int(box[5])]} {confidence:.2f}'
cv2.rectangle(image, (x1, y1), (x2, y2), (0, 255, 0), 2)
cv2.putText(image, label, (x1, y1 - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.9, (0, 255, 0), 2)
# 显示结果图像
image = image.squeeze().permute(1, 2, 0).cpu().numpy()
cv2.imshow('Pedestrian Detection', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
# 调用函数进行行人检测
image_path = 'path/to/your/image.jpg'
detect_pedestrians(image_path)
```
上述代码使用了`yolov5s`模型进行行人检测,你可以根据需要选择其他的模型版本,如`yolov5m`或`yolov5x`。请确保已安装必要的依赖库,如`torch`, `torchvision`, `PIL`, `opencv-python`等。你还需要将`image_path`替换为你要检测的图像路径。运行代码后将显示带有行人边界框和标签的图像。
希望对你有所帮助!如有其他问题,请随时提问。
相关推荐
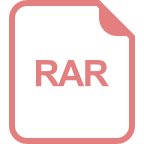
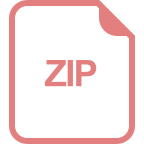
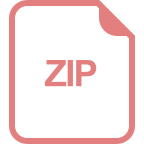












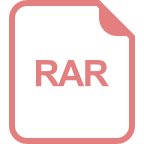
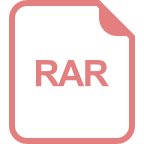
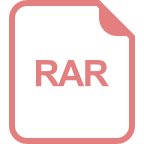