qml中的属性和函数,如何在C++中使用
时间: 2024-04-11 20:29:09 浏览: 171
在Qt中,可以通过两种方式在C++中使用QML中的属性和函数:属性绑定和信号槽连接。
属性绑定是一种将QML中的属性与C++对象的属性关联起来的方式。可以使用`QQmlContext::setContextProperty`将C++对象注册到QML上下文中,然后在QML中使用该对象的属性。在C++中修改该属性的值,QML中的属性也会相应地更新。
```cpp
// main.cpp
#include <QGuiApplication>
#include <QQmlApplicationEngine>
#include <QtQml>
class MyObject : public QObject
{
Q_OBJECT
Q_PROPERTY(QString text READ text WRITE setText NOTIFY textChanged)
public:
QString m_text;
QString text() const { return m_text; }
void setText(const QString& text) {
if (m_text != text) {
m_text = text;
emit textChanged();
}
}
signals:
void textChanged();
};
int main(int argc, char *argv[])
{
QGuiApplication app(argc, argv);
MyObject myObject;
myObject.setText("Hello from C++");
QQmlApplicationEngine engine;
engine.rootContext()->setContextProperty("myObject", &myObject);
engine.load(QUrl(QStringLiteral("qrc:/main.qml")));
return app.exec();
}
```
```qml
// main.qml
import QtQuick 2.0
Text {
text: myObject.text // 使用C++对象的属性
}
```
信号槽连接是一种在C++中响应QML中的信号和调用QML中的函数的方式。可以使用`QQmlContext::setContextProperty`将C++对象注册到QML上下文中,并在C++中使用`QObject::connect`来连接信号和槽。
```cpp
// main.cpp
#include <QGuiApplication>
#include <QQmlApplicationEngine>
#include <QtQml>
class MyObject : public QObject
{
Q_OBJECT
public slots:
void mySlot(const QString& message) {
qDebug() << "Received message from QML:" << message;
}
};
int main(int argc, char *argv[])
{
QGuiApplication app(argc, argv);
MyObject myObject;
QQmlApplicationEngine engine;
engine.rootContext()->setContextProperty("myObject", &myObject);
engine.load(QUrl(QStringLiteral("qrc:/main.qml")));
QObject::connect(&myObject, SIGNAL(mySignal(QString)), QCoreApplication::instance(), SLOT(quit()));
return app.exec();
}
```
```qml
// main.qml
import QtQuick 2.0
Button {
text: "Send Message"
onClicked: {
myObject.mySlot("Hello from QML"); // 调用C++对象的槽函数
}
}
```
通过这两种方式,可以在C++代码中获取和修改QML中的属性,以及响应QML中的信号和调用QML中的函数。这样实现了C++与QML之间的交互。
阅读全文
相关推荐
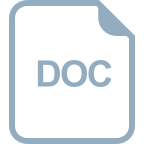
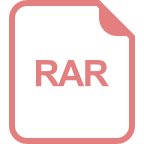
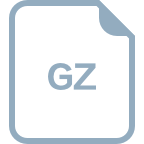






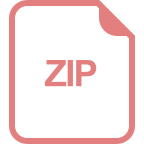
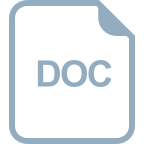
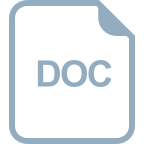




