springboot restfulapi结合spring security集成jwt身份认证
时间: 2024-12-07 18:20:41 浏览: 15
Spring Boot 结合 Spring Security 和 JWT(JSON Web Tokens)集成身份验证是一种常见的微服务安全实践。JWT 用于在客户端和服务端之间传递安全的身份信息。以下是集成步骤:
1. 添加依赖:首先,在你的`pom.xml`文件中添加Spring Security、Spring MVC以及JWT处理库的依赖,例如`jjwt`, `spring-security-oauth2-jwt`等。
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>io.jsonwebtoken</groupId>
<artifactId>jjwt</artifactId>
</dependency>
```
2. 配置Spring Security:在`SecurityConfig.java`或相应的配置类中,启用WebSecurity,设置JWT处理器,并配置授权管理器。
```java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private JwtTokenProvider jwtTokenProvider;
@Bean
public JwtAuthenticationConverter jwtAuthenticationConverter() {
return new JwtAuthenticationConverter();
}
//...
}
```
3. 创建JWT Token:当你需要生成JWT token时,可以创建一个方法,比如用户登录成功后返回带有用户名、过期时间等信息的token。
4. 自定义AuthenticationSuccessHandler:当用户通过JWT验证后,自定义这个处理器将生成的token写入到响应头中。
5. 请求拦截:在`WebMvcConfigurer`中注册JWT过滤器,检查请求头中的Authorization字段是否包含有效的JWT。
```java
@Configuration
public class JwtAutoConfiguration implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/**")
.allowedOrigins("*") // 允许跨域请求
.allowCredentials(true) // 允许发送凭据
.allowedMethods("*");
}
//其他配置...
}
```
6. 授权保护:在需要权限控制的API上使用`@PreAuthorize`注解来检查是否有访问令牌并有效。
```java
@RestController
@RequestMapping("/protected")
public class ProtectedController {
@GetMapping
@PreAuthorize("#oauth2.hasScope('read')")
public String getData() {
return "Protected data";
}
}
阅读全文
相关推荐
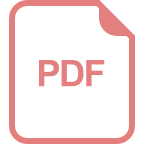
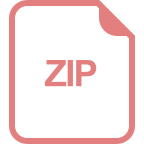
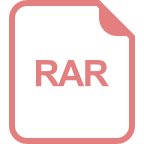

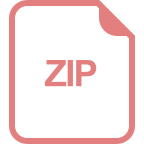
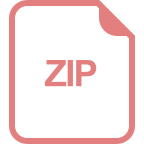
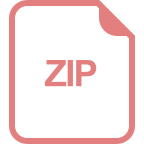
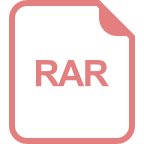
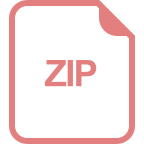
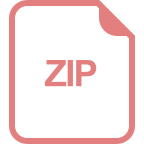
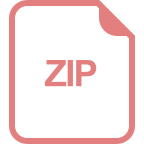
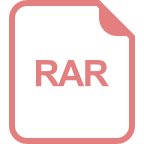
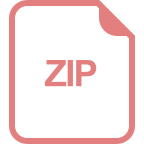
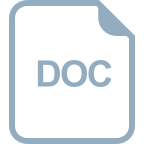
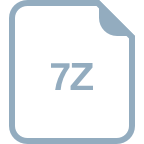
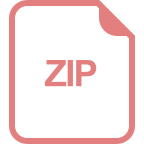
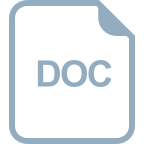
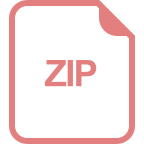
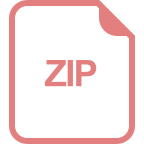