python实现RSA无填充加密
时间: 2024-09-18 13:14:36 浏览: 71
在Python中实现RSA(Rivest-Shamir-Adleman)算法的无填充(Plain Text)加密通常涉及生成密钥对、选择明文、然后直接对明文进行异或操作(如果需要的话,可以先将明文转换为大整数)。以下是基本步骤:
1. 导入必要的库:
```python
from Crypto.PublicKey import RSA
from Crypto.Cipher import PKCS1_OAEP
```
2. 生成RSA密钥对(公钥和私钥):
```python
key = RSA.generate(2048) # 生成2048位的密钥对
public_key = key.publickey()
private_key = key
```
3. 将明文(非字符串)转换为大整数(因为RSA操作在整数域内):
```python
plaintext_int = int.from_bytes(plaintext.encode(), byteorder='big')
```
4. 对明文进行无填充加密,这里使用`PKCS1_OAEP`模式:
```python
cipher_rsa = PKCS1_OAEP.new(public_key)
ciphertext = cipher_rsa.encrypt(plaintext_int)
```
5. 获得加密后的字节串,这已经是一个无填充的RSA密文。
解密时,则使用相应的私钥和类似的过程进行反向操作。
相关问题
python实现rsa算法解密
在Python中实现RSA算法的解密通常需要使用第三方库,如`pycryptodome`或`cryptography`。这里我会简述一下基本步骤:
首先,确保安装了所需的库:
```bash
pip install pycryptodome
```
接下来,创建一对RSA密钥对(包括公钥和私钥):
```python
from Crypto.PublicKey import RSA
def generate_rsa_keys(key_size=2048):
key = RSA.generate(key_size)
public_key = key.publickey().export_key()
private_key = key.export_key()
return public_key, private_key
public_key, private_key = generate_rsa_keys()
```
解密函数会接受加密后的数据以及私钥:
```python
from Crypto.Cipher import PKCS1_OAEP
import base64
def rsa_decrypt(ciphertext, private_key):
# 将私钥从字符串转换为二进制
private_key = bytes.fromhex(private_key)
# 加载私钥
rsa_cipher = PKCS1_OAEP.new(RSA.import_key(private_key))
# 解码Base64编码的密文
ciphertext_decoded = base64.b64decode(ciphertext)
# 解密数据
plaintext = rsa_cipher.decrypt(ciphertext_decoded)
return plaintext.decode('utf-8') # 如果需要文本输出,记得指定编码格式
# 使用私钥解密
encrypted_text = "..." # 假设这是加密后的数据
decrypted_text = rsa_decrypt(encrypted_text, private_key)
print("Decrypted text:", decrypted_text)
```
在这个例子中,你需要替换`encrypted_text`为你实际接收到的加密数据。注意,如果你是在网络上接收到的数据,可能还需要额外处理,比如Base64解码和可能的填充前导零。
python实现rsa加解密算法
RSA加密算法是一种非对称加密算法,可以使用Python进行实现。Python中有现成的加密库Crypto,使用它可以方便地实现RSA加密算法。需要先安装Crypto库,然后进行如下操作:
1. 生成RSA密钥对
from Crypto.PublicKey import RSA
RSA_key = RSA.generate(1024)
2. 使用公钥加密,私钥解密
# 使用公钥加密
public_key = RSA_key.publickey().exportKey()
message = b'Hello'
ciphertext = RSA.importKey(public_key).encrypt(message, None)
# 使用私钥解密
private_key = RSA_key.exportKey()
plaintext = RSA.importKey(private_key).decrypt(ciphertext)
以上是一个简单的示例,具体实现还需要考虑加解密的数据格式、填充方式等问题。
阅读全文
相关推荐
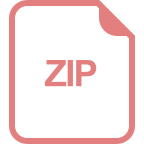
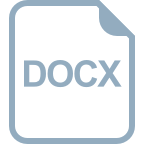
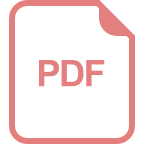













