求数组中最大值C语言
时间: 2024-10-08 14:12:00 浏览: 41
在C语言中,找到数组中的最大值通常可以使用循环结构遍历整个数组,并维护一个变量来保存当前找到的最大值。以下是简单的示例:
```c
#include <stdio.h>
int findMax(int arr[], int size) {
// 假设第一个元素就是最大值
int max = arr[0];
// 遍历数组从第二个元素开始
for (int i = 1; i < size; i++) {
// 如果当前元素大于已知的最大值,则更新最大值
if (arr[i] > max) {
max = arr[i];
}
}
return max;
}
int main() {
int numbers[] = {5, 10, 1, 8, 7};
int array_size = sizeof(numbers) / sizeof(numbers[0]);
int max_value = findMax(numbers, array_size);
printf("数组中的最大值是: %d\n", max_value);
return 0;
}
```
在这个例子中,`findMax`函数接受一个整数数组和其大小作为参数,然后返回数组中的最大值。在`main`函数里,我们创建了一个数组并调用`findMax`来找出最大值。
相关问题
输入数组求最大值c语言
以下是使用C语言输入数组求最大值的示例代码:
```c
#include <stdio.h>
int main() {
int arr[10];
int max = 0;
for (int i = 0; i < 10; i++) {
scanf("%d", &arr[i]);
if (arr[i] > max) {
max = arr[i];
}
}
printf("Max value: %d", max);
return 0;
}
```
该程序首先定义了一个长度为10的整型数组`arr`,然后通过循环和`scanf`函数依次读入数组中的10个整数。在读入的同时,使用一个变量`max`记录当前已经读入的最大值。如果读入的数比`max`大,则更新`max`的值。最后,输出`max`即为数组中的最大值。
怎么求数组最大值用C语言
使用C语言求数组最大值的方法是遍历整个数组,将每个元素与当前最大值进行比较,如果当前元素大于当前最大值,则将当前元素赋值为最大值,最后得到的最大值即为数组最大值。以下是示例代码:
int arr[] = {3, 5, 2, 8, 1, 7};
int max = arr[0];
for (int i=1; i<6; i++) {
if (arr[i] > max) {
max = arr[i];
}
}
printf("数组最大值为:%d\n", max);
阅读全文
相关推荐
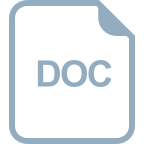
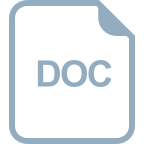
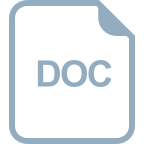



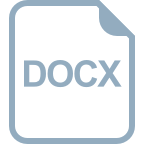
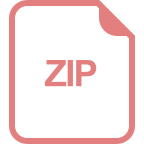






