python爬虫抓取百度贴吧
时间: 2023-11-08 07:52:09 浏览: 119
根据提供的引用内容,使用Python爬虫可以抓取百度贴吧的内容。可以通过urllib2模块发送请求并获取响应,然后解析响应内容来获取帖子标题和内容。
下面是一个示例代码:
```python
import urllib2
def crawl_tieba(url):
try:
request = urllib2.Request(url)
response = urllib2.urlopen(request)
content = response.read().decode('utf-8')
# 解析帖子标题和内容的代码
return title, content
except urllib2.URLError, e:
if hasattr(e, "reason"):
print u"连接百度贴吧失败,错误原因:", e.reason
return None
# 调用爬虫函数
url = "http://tieba.baidu.com/..."
title, content = crawl_tieba(url)
```
相关问题
python爬虫抓取百度贴吧数据
为了爬取百度贴吧数据,我们可以使用Python的requests和BeautifulSoup库。具体步骤如下:
1. 导入requests和BeautifulSoup库
```python
import requests
from bs4 import BeautifulSoup
```
2. 构造URL并发送请求
```python
url = 'https://tieba.baidu.com/f?kw=python&ie=utf-8&pn=0'
response = requests.get(url)
```
其中,kw参数指定了要爬取的贴吧名称,pn参数指定了要爬取的页数。
3. 解析HTML并提取数据
```python
soup = BeautifulSoup(response.text, 'html.parser')
post_list = soup.find_all('li', class_='j_thread_list clearfix')
for post in post_list:
title = post.find('a', class_='j_th_tit').text.strip()
author = post.find('span', class_='tb_icon_author').text.strip()
reply_num = post.find('span', class_='threadlist_rep_num').text.strip()
print('标题:', title)
print('作者:', author)
print('回复数:', reply_num)
```
其中,我们使用find_all方法找到所有的帖子,然后使用find方法找到每个帖子的标题、作者和回复数,并打印出来。
完整代码如下:
```python
import requests
from bs4 import BeautifulSoup
url = 'https://tieba.baidu.com/f?kw=python&ie=utf-8&pn=0'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
post_list = soup.find_all('li', class_='j_thread_list clearfix')
for post in post_list:
title = post.find('a', class_='j_th_tit').text.strip()
author = post.find('span', class_='tb_icon_author').text.strip()
reply_num = post.find('span', class_='threadlist_rep_num').text.strip()
print('标题:', title)
print('作者:', author)
print('回复数:', reply_num)
```
网络爬虫python百度贴吧
网络爬虫是一种自动化程序,用于从网站上抓取数据。Python作为常见的网络爬虫语言,尤其适合用于爬取百度贴吧的数据,因为其有丰富的库如BeautifulSoup、Requests和Scrapy等,可以方便地处理HTML文档并进行数据提取。
使用Python进行百度贴吧爬虫的一般步骤包括:
1. **安装依赖**:首先需要安装`requests`库用于发送HTTP请求获取网页内容,`lxml`或`BeautifulSoup4`用于解析HTML。
```bash
pip install requests beautifulsoup4
```
2. **编写代码**:
- 发送GET请求获取帖子列表页的HTML。
- 使用BeautifulSoup解析HTML,找到包含帖子链接的部分(通常在`a`标签内)。
- 对每个链接,再发送一次请求获取详细页面的内容,同样解析提取所需信息(如标题、内容等)。
以下是一个简单的示例代码片段,展示了如何获取帖子标题:
```python
import requests
from bs4 import BeautifulSoup
def get_baidu_tieba_post(url):
response = requests.get(url)
soup = BeautifulSoup(response.text, 'lxml')
title_tag = soup.find('div', {'class': 'thread-title'})
if title_tag:
title = title_tag.get_text()
print(title)
# 示例URL
url = "https://tieba.baidu.com/p/XXXXX" # 替换为实际的帖子链接
get_baidu_tieba_post(url)
```
注意:爬虫操作需遵守网站的robots.txt协议,并尊重版权,避免频繁请求导致服务器压力过大。
相关推荐
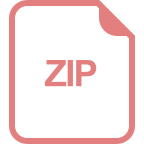
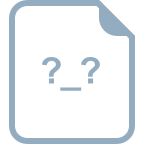











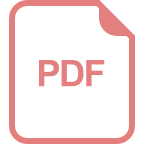
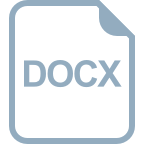
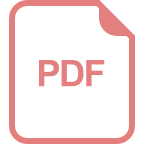