修改文件夹中图片名字,按照1,2,3等序号排列,c++代码实现,写成bat格式
时间: 2024-10-20 12:05:11 浏览: 21
在C++中,你可以使用`<filesystem>`库来操作文件和目录,但是注意Windows批处理文件(.bat)通常更适合命令行操作,而不是编程语言代码。这里是一个简单的C++程序,它可以在控制台环境中运行,用于修改文件夹中图片名称,但你需要将这个程序转换为.bat格式并添加到批处理文件中。
首先,确保你有C++环境,并且已经包含了`#include <filesystem>`头文件。然后在C++中编写如下的代码:
```cpp
#include <iostream>
#include <filesystem>
namespace fs = std::filesystem;
int main() {
// 设置待处理的图片文件夹路径
const std::string folder_path = "path_to_your_folder";
if (!fs::exists(folder_path)) {
std::cerr << "Folder not found!" << std::endl;
return 1;
}
// 获取当前目录下所有图片文件(假设都是.jpg)
auto files = fs::directory_iterator(folder_path), end;
for (auto file = files; file != end; ++file) {
if (fs::is_regular_file(file->status()) && file->path().extension() == ".jpg") {
// 获取当前图片的原名
std::string old_name = file->path().filename();
// 根据文件名提取数字,如果没有直接编号,则默认为1
int number = std::stoi(old_name.substr(0, old_name.find_last_of("_")));
// 创建新的文件名
std::string new_name = std::to_string(number + 1) + "_" + old_name.substr(old_name.find_last_of("_") + 1);
// 更改文件名
try {
fs::rename(file->path(), folder_path / new_name);
std::cout << "Renamed " << old_name << " to " << new_name << std::endl;
} catch (const fs::filesystem_error& e) {
std::cerr << "Error renaming file: " << e.what() << std::endl;
}
}
}
return 0;
}
```
请注意,这个C++程序需要手动转译成.bat格式,并可能需要相应的批处理知识来设置工作目录、添加必要的命令行参数等。批处理文件可能会更像这样:
```batch
@echo off
setlocal enabledelayedexpansion
rem Set the path to your image folder here
set FOLDER_PATH=path_to_your_folder
if not exist !FOLDER_PATH! (
echo Folder not found!
exit /b 1
)
for %%i in (!FOLDER_PATH!\*.jpg) do (
set "OLD_NAME=%%~ni"
set "NUMBER=!OLD_NAME:~0,-4!"
rem Convert name to new format and rename the file
ren "%%i" "!NUMBER!_!OLD_NAME:~-4!.jpg"
)
pause
```
记得替换`path_to_your_folder`为你实际的图片文件夹路径。
阅读全文
相关推荐
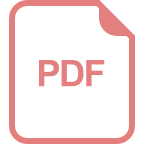
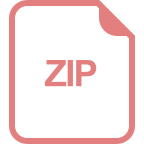
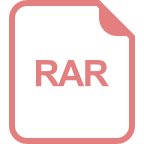
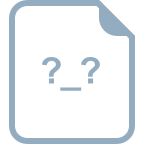
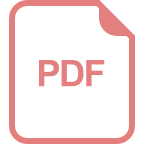
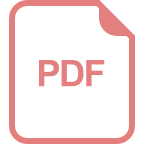
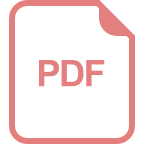
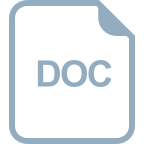
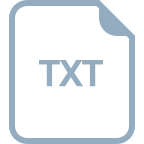
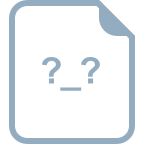
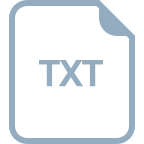
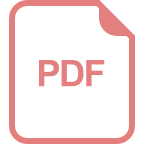
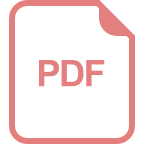
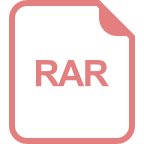