C++ WORD转std::string
时间: 2024-06-15 17:08:57 浏览: 152
在C++中,将一个WORD文档转换为std::string可以通过使用适当的库来实现。一个常用的库是Microsoft Office的COM组件,可以使用它来操作WORD文档并将其内容转换为std::string。
以下是一个简单的示例代码,演示了如何使用COM组件将WORD文档转换为std::string:
```cpp
#include <iostream>
#include <string>
#include <comutil.h>
#include <comdef.h>
#include <atlbase.h>
std::string WordToStdString(const std::wstring& filePath)
{
std::string result;
// 初始化COM组件
CoInitialize(NULL);
try {
// 创建Word应用程序对象
_ApplicationPtr pWordApp;
HRESULT hr = pWordApp.CreateInstance(__uuidof(Application));
if (FAILED(hr)) {
throw std::runtime_error("Failed to create Word application object");
}
// 打开文档
DocumentsPtr pDocs = pWordApp->Documents;
_DocumentPtr pDoc = pDocs->Open(filePath.c_str());
// 提取文档内容
_bstr_t content = pDoc->Content->Text;
// 将内容转换为std::string
result = static_cast<const char*>(content);
// 关闭文档
pDoc->Close();
// 退出Word应用程序
pWordApp->Quit();
}
catch (const _com_error& e) {
std::cerr << "COM Error: " << e.ErrorMessage() << std::endl;
}
// 释放COM资源
CoUninitialize();
return result;
}
int main()
{
std::wstring filePath = L"example.docx";
std::string content = WordToStdString(filePath);
std::cout << "Content: " << content << std::endl;
return 0;
}
```
请注意,上述示例代码使用了`_ApplicationPtr`和`_DocumentPtr`等COM组件的智能指针,这些指针会自动处理COM资源的释放,避免内存泄漏。
阅读全文
相关推荐
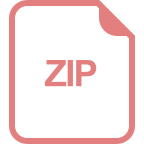
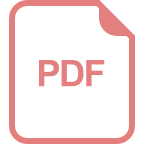

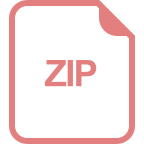
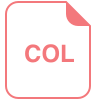
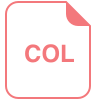
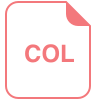
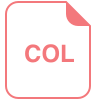
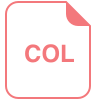

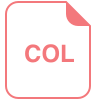
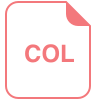
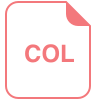
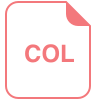
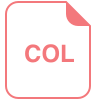
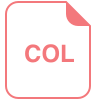
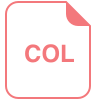
