用 C 语言 编写学生基本信息管理程序。用链表存储一个班的学生信息(最多100位学生),每个学生的信息有:学号,姓名,性别,联系电话和家庭住址。需要实现的功能有:新建学生信息链表,对学生信息进行增加、删除和查找。完整代码
时间: 2024-09-17 07:01:55 浏览: 19
在C语言中编写学生基本信息管理程序,可以使用结构体定义学生的属性,然后通过链表数据结构存储学生信息。以下是简化的代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义学生信息结构
typedef struct Student {
int id;
char name[50];
char gender[10];
char phone[20];
char address[100];
struct Student* next;
} Student;
// 创建一个新的学生节点
Student* createStudent(int id, char* name, char* gender, char* phone, char* address) {
Student* newStudent = (Student*)malloc(sizeof(Student));
newStudent->id = id;
strcpy(newStudent->name, name);
strcpy(newStudent->gender, gender);
strcpy(newStudent->phone, phone);
strcpy(newStudent->address, address);
newStudent->next = NULL;
return newStudent;
}
// 新建链表
Student* createStudentList() {
return NULL;
}
// 添加新学生到链表尾部
void addStudent(Student** head, int id, char* name, char* gender, char* phone, char* address) {
if (*head == NULL) {
*head = createStudent(id, name, gender, phone, address);
} else {
Student* current = *head;
while (current->next != NULL) {
current = current->next;
}
current->next = createStudent(id, name, gender, phone, address);
}
}
// 删除指定ID的学生
void deleteStudent(Student** head, int id) {
if (*head == NULL) return;
if ((*head)->id == id) {
Student* temp = *head;
*head = (*head)->next;
free(temp);
return;
}
Student* current = *head;
while (current->next != NULL && current->next->id != id) {
current = current->next;
}
if (current->next != NULL) {
Student* temp = current->next;
current->next = current->next->next;
free(temp);
}
}
// 查找学生
Student* findStudent(Student* head, int id) {
Student* current = head;
while (current != NULL) {
if (current->id == id) return current;
current = current->next;
}
return NULL;
}
int main() {
// 初始化链表为空
Student* studentList = createStudentList();
// 添加一些学生
addStudent(&studentList, 1001, "张三", "男", "13812345678", "北京市");
addStudent(&studentList, 1002, "李四", "女", "13987654321", "上海市");
// 打印链表中的所有学生
printStudentList(studentList);
// 删除学生
deleteStudent(&studentList, 1001);
// 再次打印链表
printStudentList(studentList);
return 0;
}
// 辅助函数,用于遍历并打印链表
void printStudentList(Student* head) {
while (head != NULL) {
printf("学号: %d, 姓名: %s, 性别: %s, 电话: %s, 地址: %s\n",
head->id, head->name, head->gender, head->phone, head->address);
head = head->next;
}
}
```
这个程序实现了创建学生列表、添加学生、删除学生和查找学生的基本功能。注意,这只是一个简化版本,实际项目可能会有更多的错误处理和边界检查。运行`main()`函数即可看到操作效果。
阅读全文
相关推荐
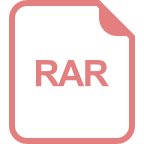
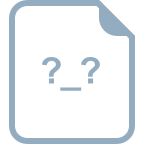
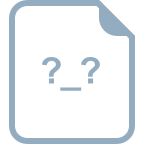
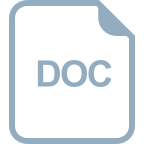



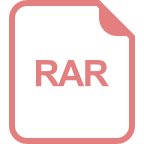
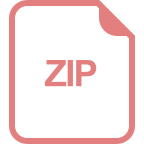
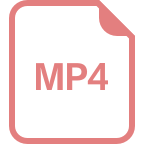
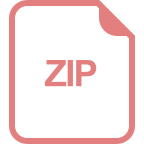
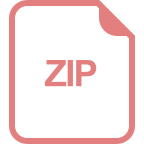
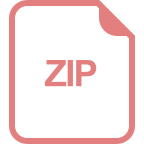