C++中怎么输入分数并化成浮点数比较
时间: 2024-12-12 12:21:54 浏览: 7
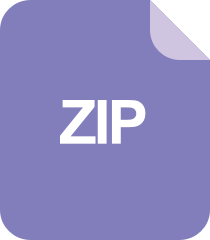
Fraction:用于使用分数而不是浮点类型的 C++ 库
在C++中,你可以通过获取用户的输入,并使用`std::cin`从控制台读取分数,然后将其转换为浮点数进行比较。这里是一个简单的步骤示例:
```cpp
#include <iostream>
#include <string>
// 函数用于将字符串分数转换为浮点数
double convertToFloat(const std::string& score) {
double result;
bool success = std::stod(score, &result);
if (success) {
return result;
} else {
throw std::invalid_argument("Invalid score format");
}
}
int main() {
try {
std::cout << "请输入一个分数(如:3/4):" << std::endl;
std::string inputScore;
std::getline(std::cin, inputScore);
double floatScore = convertToFloat(inputScore);
// 现在可以进行浮点数比较
double anotherScore; // 假设这是另一个用户输入的分数
std::cin >> anotherScore;
if (floatScore > anotherScore) {
std::cout << "第一个分数大于第二个分数" << std::endl;
} else if (floatScore < anotherScore) {
std::cout << "第一个分数小于第二个分数" << std::endl;
} else {
std::cout << "两个分数相等" << std::endl;
}
} catch (const std::invalid_argument& e) {
std::cerr << "错误: " << e.what() << std::endl;
}
return 0;
}
```
在这个例子中,我们首先获取用户的输入作为字符串,然后尝试使用`std::stod`函数将其转换为浮点数。如果输入格式不是有效的分数,就会抛出一个异常。
阅读全文
相关推荐
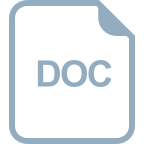
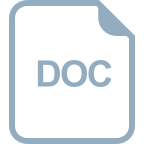
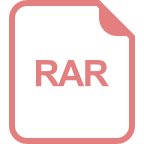
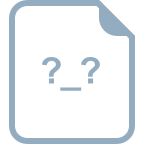
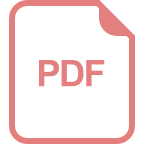
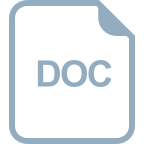
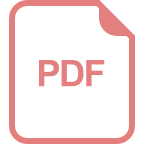
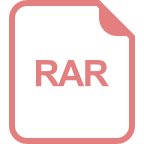
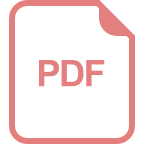
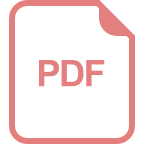
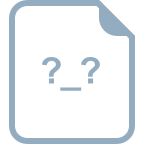
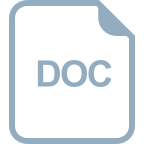
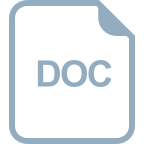