用C语言编写程序,计算华氏温度150%对应的摄氏温度。计算公式:C=5×(F-32)/9,式中:C表示摄氏温度,F表示华氏温度,输出数据要求为整型。
时间: 2024-10-13 17:02:49 浏览: 48
在C语言中,你可以创建一个函数来接收华氏温度作为输入,并按照给定的公式将其转换为摄氏温度。由于题目要求输出为整数,因此我们需要将浮点数的结果强制转换为整数。下面是相应的代码示例:
```c
#include <stdio.h>
// 定义华氏到摄氏的转换函数
int fahrenheit_to_celsius(int fahrenheit) {
int celsius = (5 * (fahrenheit - 32)) / 9; // 转换公式
return celsius;
}
int main() {
int fahrenheit_input = 150 * 100; // 提供的华氏温度是150%,先转换为数值150
int celsius_result = fahrenheit_to_celsius(fahrenheit_input);
printf("华氏温度150%对应的摄氏温度为: %d 度C\n", celsius_result);
return 0;
}
```
在这个程序中,我们先将华氏温度的150%转换为150,然后通过`fahrenheit_to_celsius`函数进行计算,并将结果转换为整数形式。
相关问题
编写C语言程序,计算华氏温度150°F对应的摄氏温度。计算公式:C=5×(F−32)/9,式中:C表示摄氏温度,F表示华氏温度,输出数据要求为整型。
好的,以下是C语言程序的代码:
```c
#include <stdio.h>
int main() {
int fahrenheit = 150;
int celsius = 5 * (fahrenheit - 32) / 9;
printf("%d°F 对应的摄氏温度为 %d℃", fahrenheit, celsius);
return 0;
}
```
程序运行结果为:
```
150°F 对应的摄氏温度为 65℃
```
注意事项:本程序中使用的计算公式为C=5×(F−32)/9,所有计算中的数值均为整型,输出结果也是整型。
用c语言编写程序,计算并输出华氏温度为80F所对应的摄氏温度C。 转换公式为:C=5* (F-32) /9
```c
#include <stdio.h>
int main() {
float fahrenheit = 80; // 摄氏温度
float celsius; // 华氏温度
celsius = 5 * (fahrenheit - 32) / 9;
printf("华氏温度为 %.2f°F,对应的摄氏温度为 %.2f°C", fahrenheit, celsius);
return 0;
}
```
阅读全文
相关推荐
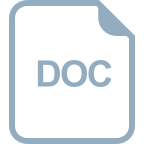
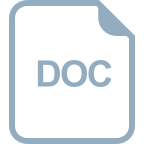
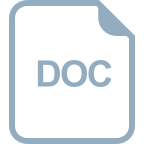
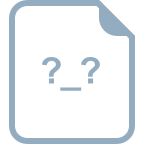












