c++ 刷题常用函数
时间: 2023-09-26 14:13:48 浏览: 171
常用的C++刷题函数包括binary_search()和find()。
binary_search()函数用于在已排序的区间中查找指定元素。它返回一个bool类型的值,如果找到了指定元素,则返回true;否则返回false。
下面是一个使用binary_search()函数的例子:
```cpp
#include <iostream>
#include <algorithm>
#include <vector>
using namespace std;
int main() {
int a[7] = {1, 2, 3, 4, 5, 6, 7};
bool haselem = binary_search(a, a + 7, 4);
cout << "haselem: " << haselem << endl;
vector<int> myvector{4, 5, 3, 1, 2};
bool haselem2 = binary_search(myvector.begin(), myvector.end(), 3);
cout << "haselem2: " << haselem2 << endl;
return 0;
}
```
另一个常用的函数是find()函数,它用于在区间中查找指定元素。如果查找成功,则返回指向第一个目标元素的迭代器;如果查找失败,则返回指向区间末尾的迭代器。
下面是一个使用find()函数的例子:
```cpp
#include <iostream>
#include <algorithm>
#include <vector>
using namespace std;
int main() {
char stl[] = "<http://c.biancheng.net/stl/>";
char *p = find(stl, stl + strlen(stl), 'c');
if (p != stl + strlen(stl)) {
cout << p << endl;
}
vector<int> myvector{10, 20, 30, 40, 50};
vector<int>::iterator it = find(myvector.begin(), myvector.end(), 30);
if (it != myvector.end()) {
cout << "查找成功: " << *it << endl;
} else {
cout << "查找失败" << endl;
}
return 0;
}
```
阅读全文
相关推荐
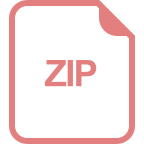
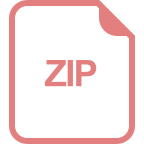


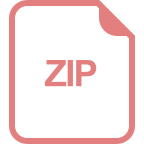
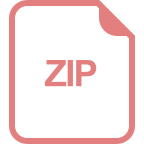
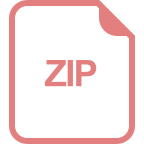
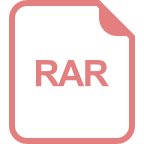
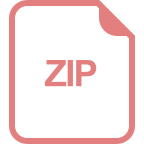
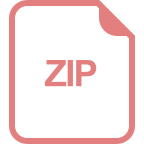
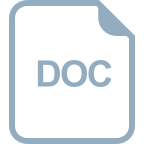
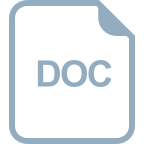
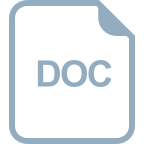
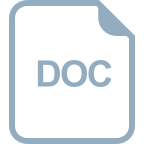
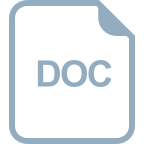
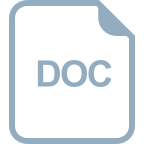
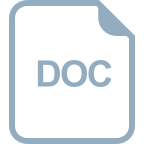
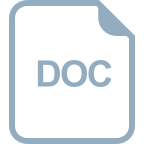
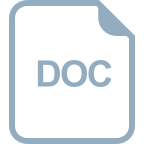