C++生成供Linux Shaow使用的ASH512加密密码
时间: 2024-09-10 19:15:30 浏览: 63
在C++中,要为Linux Shadow系统生成SHA512加密的密码,你可以使用标准库中的`<openssl/sha.h>`来计算SHA512哈希,然后将结果转换为暗文格式,即Unix/Linux的Shadow文件中的密码存储形式。首先,确保安装了OpenSSL库,并且在编译时链接了相应的头文件和库。
以下是一个简单的步骤说明:
1. 引入必要的头文件:
```cpp
#include <openssl/sha.h>
#include <string>
```
2. 定义一个函数来计算SHA512哈希:
```cpp
std::string sha512_hash(const std::string& password) {
unsigned char hash[SHA512_DIGEST_LENGTH];
SHA512((unsigned char*)password.c_str(), password.length(), hash);
std::stringstream ss;
for (size_t i = 0; i < SHA512_DIGEST_LENGTH; ++i) {
ss << std::hex << static_cast<int>(hash[i]);
}
return ss.str();
}
```
这个函数接受一个字符串作为输入,将其转换为二进制数据,然后使用SHA512算法计算哈希值,最后把哈希值转成十六进制字符串。
3. 转换为暗文格式(64个字符):
```cpp
std::string to_shadow_format(const std::string &hashed_password) {
if (hashed_password.size() > 64) {
hashed_password.resize(64); // Truncate to the maximum length allowed in Shadow format
} else if (hashed_password.size() < 64) {
hashed_password.append(std::string(64 - hashed_password.size(), '*')); // Pad with '*' characters
}
return hashed_password;
}
// 使用例子
std::string user_password = "your_password";
std::string shadow_password = to_shadow_format(sha512_hash(user_password));
```
现在`shadow_password`变量就是经过SHA512加密并转换为暗文格式的密码了。
阅读全文
相关推荐





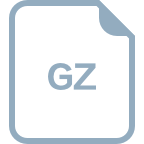











