python画散点图拟合一元四次曲线
时间: 2024-10-05 08:02:09 浏览: 33
在Python中,可以使用matplotlib库来创建散点图,并通过numpy库计算一元四次多项式来拟合数据。首先,你需要导入所需的库:
```python
import matplotlib.pyplot as plt
import numpy as np
from scipy.optimize import curve_fit
```
然后,假设你已经有了x值的列表(表示自变量)和y值的列表(表示因变量),可以用以下步骤绘制散点图并进行拟合:
1. 定义一个四次函数模型(例如:f(x) = ax^4 + bx^3 + cx^2 + dx + e):
```python
def quartic_func(x, a, b, c, d, e):
return a * x**4 + b * x**3 + c * x**2 + d * x + e
```
2. 使用`curve_fit`函数从数据中找到最优的五参数(a, b, c, d, e):
```python
# 假设你的数据是 x_data, y_data
popt, _ = curve_fit(quartic_func, x_data, y_data)
```
3. 绘制散点图和拟合曲线:
```python
# 创建一个新的x值范围进行拟合曲线显示
x_range = np.linspace(min(x_data), max(x_data), 100)
# 计算拟合曲线对应的y值
y_fit = quartic_func(x_range, *popt)
# 创建图表
plt.figure()
plt.scatter(x_data, y_data, label='Data points')
plt.plot(x_range, y_fit, 'r', linewidth=2, label='Fitted curve')
# 添加标签、标题和图例
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Scatter plot with quartic fit')
plt.legend()
# 显示图形
plt.show()
```
阅读全文
相关推荐
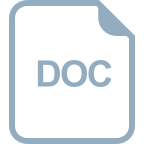
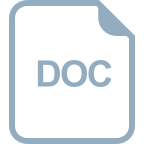
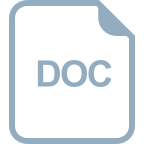
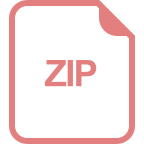
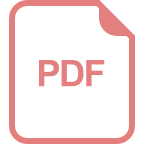
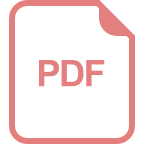
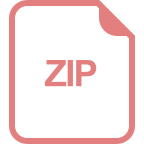
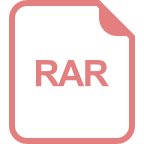
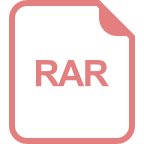
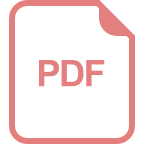
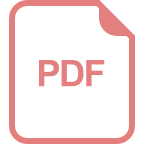
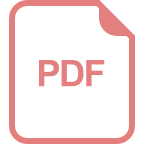
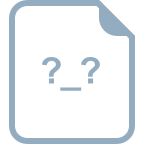
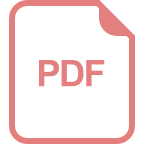
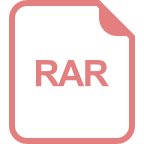
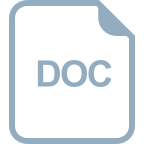
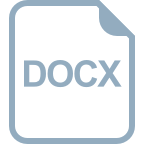