vs2019中使用C++根据年月日命名文件名
时间: 2024-09-21 14:03:34 浏览: 49
在Visual Studio 2019中,如果你想根据当前的日期来命名C++项目中的文件,可以使用标准库中的`<ctime>`和`<iomanip>`来获取日期,并将其格式化为字符串。下面是一个简单的例子,说明如何创建一个包含当前日期的文件名:
```cpp
#include <iostream>
#include <fstream>
#include <ctime>
#include <sstream>
#include <filesystem> // C++17引入的新特性,用于处理文件和目录
namespace fs = std::filesystem;
void createFileNameWithDate(const std::string& path, std::string& fileName) {
// 获取当前时间(本地时间)
time_t now = time(0);
// 将时间转换为tm结构体以便格式化
tm* localTime = localtime(&now);
// 创建日期字符串,格式化为 "YYYYMMDD"
std::ostringstream oss;
oss << std::setfill('0') << std::setw(4) << localTime->tm_year + 1900; // 年份
oss << std::setfill('0') << std::setw(2) << localTime->tm_mon + 1; // 月份
oss << std::setfill('0') << std::setw(2) << localTime->tm_mday; // 日期
// 组合完整的文件名(例如:path/year/month/day.txt)
fileName = path + "/" + oss.str() + ".txt";
}
int main() {
std::string basePath = "C:/MyFiles/"; // 文件存放的基本路径
std::string currentDateFileName;
// 创建一个当前日期的文件名
createFileNameWithDate(basePath, currentDateFileName);
try {
// 检查文件是否已经存在,如果不存在就创建
if (!fs::exists(currentDateFileName)) {
std::ofstream outFile(currentDateFileName);
if (outFile) {
outFile << "This file was created on " << currentDateFileName << std::endl;
outFile.close();
} else {
std::cerr << "Failed to create file: " << currentDateFileName << std::endl;
}
} else {
std::cout << "File already exists: " << currentDateFileName << std::endl;
}
} catch (const fs::filesystem_error& e) {
std::cerr << "Error creating file: " << e.what() << std::endl;
}
return 0;
}
```
这个程序会创建一个名为`YYYYMMDD.txt`的文件,其中包含创建该文件的确切日期。如果文件已存在,它将不会再次创建。
阅读全文
相关推荐
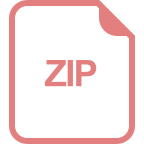
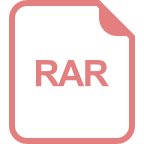

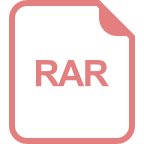
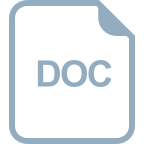
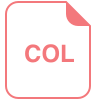

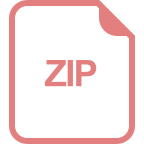
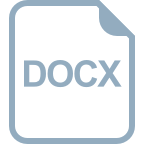