使用面向对象的思想编写一个计算机类,可以实现两个整数的加减乘除,测试类中创建计算机类的对象并做测试
时间: 2024-12-22 21:12:23 浏览: 6
面向对象编程中,我们可以设计一个名为`Computer`的类,它包含整数运算的功能。这个类通常会有一个私有变量`private int value`存储数值,并提供四个公共方法`add`, `subtract`, `multiply`, 和 `divide`来进行相应的运算。下面是简单的实现:
```java
public class Computer {
private int value;
// 构造函数
public Computer(int initialValue) {
this.value = initialValue;
}
// 加法方法
public Computer add(int num) {
value += num;
return this;
}
// 减法方法
public Computer subtract(int num) {
value -= num;
return this;
}
// 乘法方法
public Computer multiply(int num) {
value *= num;
return this;
}
// 除法方法 (这里假设除数非零)
public Computer divide(int num) {
if (num != 0) {
value /= num;
} else {
throw new ArithmeticException("Cannot divide by zero");
}
return this;
}
// 获取当前值
public int getValue() {
return value;
}
}
// 测试类
public class ComputerTest {
public static void main(String[] args) {
Computer computer = new Computer(10); // 创建计算机实例
// 测试加法
computer.add(5);
System.out.println("After addition: " + computer.getValue());
// 测试减法
computer.subtract(3);
System.out.println("After subtraction: " + computer.getValue());
// 测试乘法
computer.multiply(2);
System.out.println("After multiplication: " + computer.getValue());
// 测试除法
try {
computer.divide(2);
System.out.println("After division: " + computer.getValue());
} catch (ArithmeticException e) {
System.out.println(e.getMessage());
}
}
}
```
在这个例子中,测试类`ComputerTest`展示了如何创建`Computer`对象并进行操作。运行这个程序将显示每次运算后的结果。
阅读全文
相关推荐
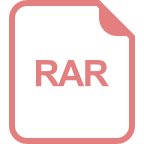
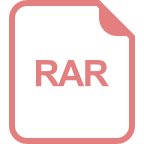
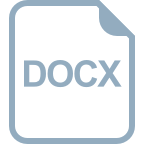







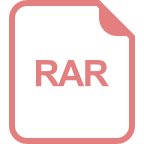
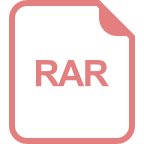
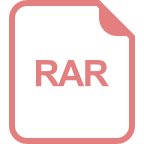
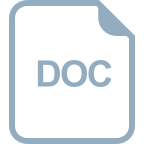
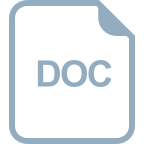
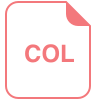

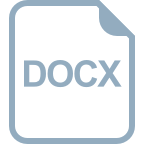