实现webservice的几种方法python
时间: 2024-06-01 15:04:09 浏览: 25
以下是三种实现Python WebService的方法:
1.使用Flask框架实现Python WebService:
```python
from flask import Flask
from spyne import Application, rpc, ServiceBase, Integer, Unicode
from spyne.protocol.soap import Soap11
from spyne.server.wsgi import WsgiApplication
app = Flask(__name__)
class HelloWorldService(ServiceBase):
@rpc(Integer, Integer, _returns=Unicode)
def say_hello(ctx, a, b):
return "Sum of {} and {} is {}".format(a, b, str(a + b))
soap_app = Application([HelloWorldService], 'spyne.examples.hello.soap',
in_protocol=Soap11(validator='lxml'),
out_protocol=Soap11())
@app.route('/hello', methods=['POST'])
def hello():
response = soap_app(spyne_request.data)
return response
if __name__ == '__main__':
app.run()
```
2.使用Django框架实现Python WebService:
```python
from django.views.decorators.csrf import csrf_exempt
from spyne import Application, rpc, ServiceBase, Integer, Unicode
from spyne.protocol.soap import Soap11
from spyne.server.django import DjangoApplication
class HelloWorldService(ServiceBase):
@rpc(Integer, Integer, _returns=Unicode)
def say_hello(ctx, a, b):
return "Sum of {} and {} is {}".format(a, b, str(a + b))
soap_app = Application([HelloWorldService], 'spyne.examples.hello.soap',
in_protocol=Soap11(validator='lxml'),
out_protocol=Soap11())
@csrf_exempt
def soap(request):
django_application = DjangoApplication(soap_app)
response = django_application(request)
return response
```
3.将Python WebService部署到Docker容器中:
```python
from spyne import Application, rpc, ServiceBase, Integer, Unicode
from spyne.protocol.soap import Soap11
from spyne.server.wsgi import WsgiApplication
class HelloWorldService(ServiceBase):
@rpc(Integer, Integer, _returns=Unicode)
def say_hello(ctx, a, b):
return "Sum of {} and {} is {}".format(a, b, str(a + b))
soap_app = Application([HelloWorldService], 'spyne.examples.hello.soap',
in_protocol=Soap11(validator='lxml'),
out_protocol=Soap11())
wsgi_application = WsgiApplication(soap_app)
if __name__ == '__main__':
from wsgiref.simple_server import make_server
server = make_server('0.0.0.0', 8000, wsgi_application)
server.serve_forever()
```
相关推荐
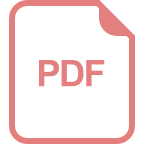
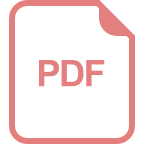
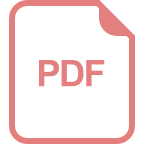














