如何统计英文句子中的每个字母频率?
时间: 2024-10-15 12:12:45 浏览: 28
统计英文句子中每个字母的频率通常可以通过以下步骤完成:
1. **文本预处理**:首先将英文句子转换为小写,以便区分大小写字母,同时移除所有非字母字符,只保留字母。
```python
sentence = "your sample sentence"
sentence = sentence.lower()
sentence = ''.join(e for e in sentence if e.isalpha())
```
2. **创建字典**:使用Python的`collections.Counter`类,它可以方便地计算元素及其出现次数。
```python
from collections import Counter
letter_counts = Counter(sentence)
```
3. **获取结果**:最后,你可以遍历字典得到每个字母及其对应的频率。
```python
for letter, count in sorted(letter_counts.items()):
print(f"{letter}: {count}")
```
这样就会按字母顺序列出每种字母以及它们在句子中出现的次数。
相关问题
怎样编写一个简单的程序来统计英文单词中的字母频率?
编写一个简单的英文单词字母频率统计程序,可以按照以下步骤:
1. **数据预处理**:首先读取英文文本,可以是一个字符串或文件内容,然后将其分割成单词列表,一般会移除标点符号和空格。
```python
import re
text = "Your English text here"
words = re.findall(r'\b\w+\b', text.lower()) # 将所有单词转换为小写并分割
```
2. **创建频率字典**:初始化一个空的字典(如Python的`collections.Counter`),用于记录每个字母及其出现次数。
```python
from collections import Counter
letter_counts = Counter()
for word in words:
letter_counts.update(word)
```
3. **输出结果**:最后遍历字典,打印出每个字母及其频率。
```python
for letter, count in letter_counts.items():
print(f"'{letter}': {count}")
```
这样就得到了一个简单英文单词字母频率的统计。
C语言中如何手动统计字母频率?
在C语言中手动统计字母频率通常涉及到字符数组、计数器数组以及遍历输入字符串的操作。以下是简单的步骤:
1. 定义一个大小为26的数组(因为英文字母有26个),每个元素代表对应字母的频率,初始化为0。例如:
```c
int letter_freq[26] = {0};
```
2. 创建一个循环来读取输入的字符串,并检查每个字符是否为英文字母(忽略空格、标点和其他非字母字符)。可以使用ASCII码表来判断:
```c
while (input_char != '\0') {
if ('a' <= input_char && input_char <= 'z' || 'A' <= input_char && input_char <= 'Z') {
// 对大写字母转换为小写,以便统一处理
if (input_char >= 'A' && input_char <= 'Z') {
input_char += 'a' - 'A';
}
letter_freq[input_char - 'a']++;
}
input_char = getchar();
}
```
3. 循环结束后,`letter_freq`数组的每个元素就记录了相应字母的频率。
4. 如果需要,你可以打印出这个数组,显示字母及其频率。例如:
```c
for (int i = 0; i < 26; i++) {
printf("%c appears %d times.\n", 'a' + i, letter_freq[i]);
}
```
阅读全文
相关推荐






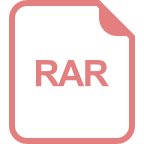










