python个人项目成果
时间: 2023-12-05 13:41:39 浏览: 139
很高兴听到你已经学习了一个月的Python,以下是一些关于Python个人项目成果的介绍:
1. 一个简单的计算器
```python
def add(x, y):
return x + y
def subtract(x, y):
return x - y
def multiply(x, y):
return x * y
def divide(x, y):
return x / y
print("Select operation.")
print("1.Add")
print("2.Subtract")
print("3.Multiply")
print("4.Divide")
choice = input("Enter choice(1/2/3/4):")
num1 = float(input("Enter first number: "))
num2 = float(input("Enter second number: "))
if choice == '1':
print(num1,"+",num2,"=", add(num1,num2))
elif choice == '2':
print(num1,"-",num2,"=", subtract(num1,num2))
elif choice == '3':
print(num1,"*",num2,"=", multiply(num1,num2))
elif choice == '4':
print(num1,"/",num2,"=", divide(num1,num2))
else:
print("Invalid input")
```
2. 一个简单的猜数字游戏
```python
import random
number = random.randint(1, 10)
guess = int(input("Guess a number between 1 and 10: "))
while guess != number:
guess = int(input("Wrong. Guess again: "))
print("You guessed it! The number was", number)
```
3. 一个简单的文本冒险游戏
```python
print("Welcome to the game!")
name = input("What is your name? ")
age = int(input("What is your age? "))
health = 10
if age >= 18:
print("You are old enough to play!")
wants_to_play = input("Do you want to play? ").lower()
if wants_to_play == "yes":
print("You are starting with", health, "health.")
print("Let's play!")
left_or_right = input("First choice... Left or Right? ").lower()
if left_or_right == "left":
ans = input("Nice, you follow the path and reach a lake... Do you swim across or go around? ").lower()
if ans == "around":
print("You went around and reached the other side of the lake.")
elif ans == "swim":
print("You managed to get across, but lost 5 health.")
health -= 5
ans = input("You notice a house and a river. Which do you go to? ").lower()
if ans == "house":
print("You go to the house and are greeted by the owner... He doesn't like you and you lose 5 health.")
health -= 5
if health <= 0:
print("You now have 0 health and you lost the game...")
else:
print("You have survived... You win!")
else:
print("You fell in the river and lost...")
else:
print("You fell down and lost...")
else:
print("Goodbye...")
else:
print("You are not old enough to play...")
```
阅读全文
相关推荐
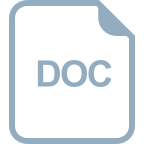
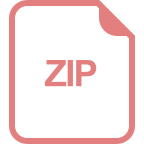
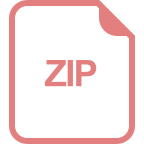
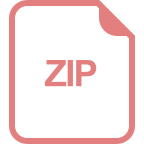
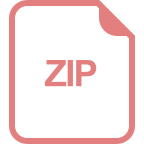
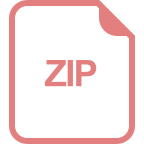
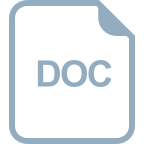
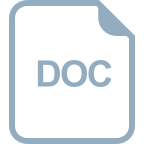
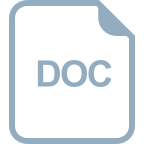
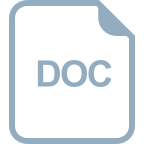
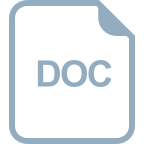
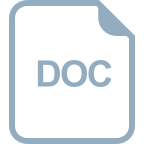
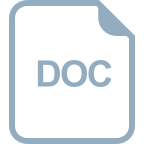
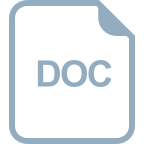
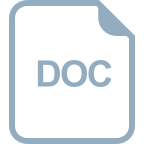
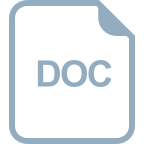