Writing a 4-lane mipi data alignment module in verilog
时间: 2024-04-11 19:26:23 浏览: 143
Sure! Here's an example of a 4-lane MIPI data alignment module written in Verilog:
```verilog
module mipi_data_alignment (
input [31:0] data_in,
input clk,
input rst,
output reg [31:0] data_out
);
reg [31:0] shift_reg [0:3];
always @(posedge clk or posedge rst) begin
if (rst) begin
for (int i = 0; i < 4; i = i + 1) begin
shift_reg[i] <= 32'b0;
end
end else begin
for (int i = 0; i < 4; i = i + 1) begin
shift_reg[i] <= {shift_reg[i][30:0], data_in[i*8 +: 8]};
end
end
end
always @(posedge clk) begin
if (rst) begin
data_out <= 32'b0;
end else begin
for (int i = 0; i < 4; i = i + 1) begin
data_out[i*8 +: 8] <= shift_reg[i][31:24];
end
end
end
endmodule
```
In this module, `data_in` is a 32-bit input representing the MIPI data for the four lanes, `clk` is the clock signal, `rst` is the reset signal, and `data_out` is the aligned output data for the four lanes.
The array `shift_reg[0:3]` consists of four 32-bit shift registers, each corresponding to one lane. On each rising edge of the clock (`clk`), the data for each lane is shifted left by eight bits, and the new input data for each lane is concatenated to the rightmost eight bits of the corresponding shift register. This aligns the incoming MIPI data stream for each lane.
The aligned output data for each lane is updated on each rising edge of the clock based on the current values of the shift registers.
Remember to customize this code according to your specific requirements and the MIPI protocol specifications you are working with.
阅读全文
相关推荐
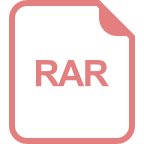
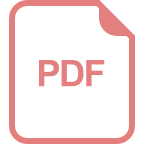
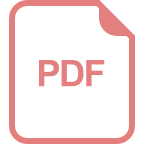















