python3D爱心代码高级
时间: 2024-11-05 12:03:53 浏览: 61
在Python中创建3D爱心形状通常需要使用一些图形库,如Pygame、PIL中的ImageDraw模块或者是更专业的3D建模库如PyOpenGL或VTK。由于这里是一个文本环境,我会给你提供一种简单的2D爱心示例,然后你可以尝试将其转换成3D。
```python
from PIL import Image, ImageDraw
# 创建一个新的空白图像
image = Image.new('RGB', (400, 400), 'white')
draw = ImageDraw.Draw(image)
# 定义心形的两个半边
def draw_half_heart(x, y, size):
draw.polygon([(x - size, y + size), (x + size, y + size), (x, y)], fill='red')
# 绘制整个爱心
half_size = image.height / 6
draw_half_heart(image.width // 2, image.height // 2, half_size)
draw_half_heart(image.width // 2, image.height // 2, half_size * 1.5)
# 显示或保存图片
image.show()
```
如果你想将这个2D爱心变成3D,你需要使用3D图形库,并将爱心视为一个面片,通过旋转和平移操作使其呈现立体效果。这可能会涉及到三角形网格渲染和光照计算,适合有一定3D图形知识的开发者。
相关问题
python3D粒子爱心代码高级
Python中创建一个3D粒子爱心的效果可以通过使用多种库来实现,其中比较高级的方式可能涉及使用`matplotlib`库中的`mplot3d`模块来绘制3D图形,以及可能使用`numpy`库来处理数学运算。以下是一个简化的示例代码,用于创建一个3D粒子爱心的效果:
```python
import numpy as np
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
# 创建一个新的figure
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
# 定义爱心形状的参数方程
t = np.linspace(0, 2 * np.pi, 100)
x = 16 * np.sin(t)**3
y = 13 * np.cos(t) - 5 * np.cos(2*t) - 2 * np.cos(3*t) - np.cos(4*t)
z = np.linspace(-1, 1, 100)
X, Z = np.meshgrid(x, z)
Y, Z = np.meshgrid(y, z)
# 为了增加粒子效果,我们可以对X, Y, Z进行轻微的随机扰动
X += np.random.normal(0, 0.01, X.shape)
Y += np.random.normal(0, 0.01, Y.shape)
Z += np.random.normal(0, 0.01, Z.shape)
# 使用scatter方法绘制3D粒子爱心
ax.scatter(X, Y, Z, c='r', marker='o')
# 设置图形标题和坐标轴标签
ax.set_title("3D Particles Heart Shape")
ax.set_xlabel("X axis")
ax.set_ylabel("Y axis")
ax.set_zlabel("Z axis")
# 显示图形
plt.show()
```
这段代码首先定义了爱心的参数方程,然后通过`scatter`方法在3D空间中绘制出爱心形状的粒子效果。你可以调整`np.linspace`和`np.random.normal`的参数来改变粒子的密度和分布。
python爱心代码高级3d
Python爱心代码是一种简单而美观的代码,它可以用几行Python代码来生成一个3D爱心图案。下面是一个Python爱心代码的示例:
```
import math
def heart_3d(x, y, z):
return (math.sin(x) * math.cos(z) * math.exp(abs(1 - (math.sqrt(x ** 2 + y ** 2 + z ** 2) / math.pi))) +
(math.sin(y) * math.cos(z) * math.exp(abs(1 - (math.sqrt(x ** 2 + y ** 2 + z ** 2) / math.pi)))) / 2.5
for i in range(-20, 20):
s = ""
for j in range(-50, 50):
if heart_3d(i * 0.1, 0, j * 0.1) <= 0.3:
s += "*"
else:
s += " "
print(s)
```
这段代码使用了数学函数和循环语句来生成一个3D爱心图案。在程序中,函数 `heart_3d` 计算出给定坐标 `(x,y,z)` 的爱心形状值,而主循环则使用该函数来绘制图案。最后,将输出的结果打印到控制台上。
阅读全文
相关推荐
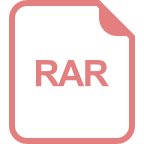
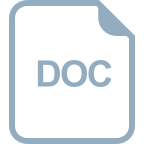
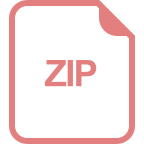
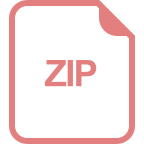
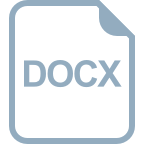
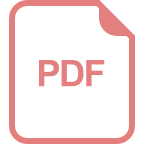
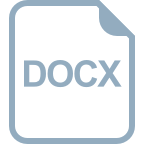
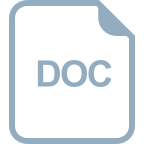
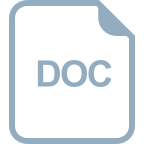
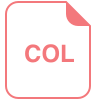



